用c语言编写一个函数,从控制台接受三个参数,一个字符和两个整数,字符参数是待打印的字符,第一个整数指定一行中打印字符的次数,第二个整数指定打印指定字符的行数
时间: 2024-02-28 13:55:56 浏览: 109
以下是用 C 语言编写的函数,它接受三个参数,其中第一个是字符,第二个是整数,表示每行需要打印的字符次数,第三个是整数,表示需要打印的行数。函数会在控制台输出指定字符,满足每行打印指定字符次数,打印指定行数。
```c
#include <stdio.h>
void print_chars(char c, int n, int m) {
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
printf("%c", c);
}
printf("\n");
}
}
int main() {
char c;
int n, m;
printf("请输入待打印的字符:");
scanf("%c", &c);
printf("请输入每行打印的次数:");
scanf("%d", &n);
printf("请输入打印的行数:");
scanf("%d", &m);
print_chars(c, n, m);
return 0;
}
```
你可以在控制台输入待打印的字符、每行打印的次数和需要打印的行数,该程序会调用 `print_chars` 函数打印指定字符。
相关问题
用c语言编写一个函数,由实参传来一个字符串,统计此字符串中字母、数字、空格和其他字符的个数,在主函数中输入字符串以及输出上述的结果
可以使用 C 语言来编写一个函数来统计字符串中字母、数字、空格和其他字符的个数。以下是一个示例函数:
```c
#include <stdio.h>
#include <ctype.h>
void count_chars(char *str, int *letters, int *digits, int *spaces, int *others) {
while (*str) {
if (isalpha(*str)) {
(*letters)++;
} else if (isdigit(*str)) {
(*digits)++;
} else if (isspace(*str)) {
(*spaces)++;
} else {
(*others)++;
}
str++;
}
}
int main() {
char str[100];
int letters = 0, digits = 0, spaces = 0, others = 0;
printf("请输入一个字符串:");
fgets(str, sizeof(str), stdin);
count_chars(str, &letters, &digits, &spaces, &others);
printf("字母个数:%d\n", letters);
printf("数字个数:%d\n", digits);
printf("空格个数:%d\n", spaces);
printf("其他字符个数:%d\n", others);
return 0;
}
```
在上面的示例中,`count_chars` 函数接受一个字符串指针和四个整数指针作为参数,分别用于存储字母、数字、空格和其他字符的个数。函数中使用了 C 语言中的 `isalpha`、`isdigit` 和 `isspace` 函数来判断当前字符属于哪一类。在主函数中,我们通过 `fgets` 函数从控制台读入一个字符串,并将其传递给 `count_chars` 函数进行统计。最后,我们输出了各个类别的字符个数。
用C语言写一个代码:【问题描述】 编写一个函数strrindex(s,t),用于返回字符串t在字符串s中最右边出现的位置.该位置从0开始计数,如果s中不含有t,那么返回-1;在你编写的程序中,使用strrindex(s,t)函数,输入t,s,输出t在s最右边的位置. 【输入形式】 控制台分行输入字符串s,t. 【输出形式】 控制台输出一个整数,是t在s最右边出现的位置.
下面是用C语言编写的strrindex函数代码:
```c
#include <stdio.h>
#include <string.h>
int strrindex(char s[], char t[]) {
int i, j, k, pos = -1;
for (i = 0; s[i] != '\0'; i++) {
for (j = i, k = 0; t[k] != '\0' && s[j] == t[k]; j++, k++);
if (k > 0 && t[k] == '\0') {
pos = i;
}
}
return pos;
}
int main() {
char s[100], t[100];
printf("请输入字符串s:");
fgets(s, 100, stdin);
printf("请输入字符串t:");
fgets(t, 100, stdin);
s[strlen(s) - 1] = '\0'; // 去除fgets函数读入的换行符
t[strlen(t) - 1] = '\0';
int pos = strrindex(s, t);
if (pos >= 0) {
printf("%s在%s中最右边出现的位置是:%d\n", t, s, pos);
} else {
printf("%s不在%s中出现\n", t, s);
}
return 0;
}
```
在该程序中,我们定义了一个名为strrindex的函数,用于返回字符串t在字符串s中最右边出现的位置。该函数的实现过程为:先使用两个循环分别遍历s和t,当s中的某个子串与t相同时,记录下该子串的位置pos。最后返回pos即可。如果s中不含有t,则返回-1。
在主函数中,我们先使用fgets函数从输入流中读入字符串s和t,并去除最后的换行符。然后调用strrindex函数求出t在s中的最右边位置,并根据pos的值输出相应的信息。
希望能够帮到您!
阅读全文
相关推荐
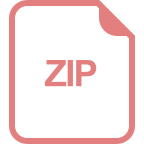







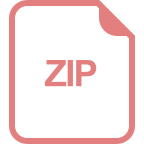
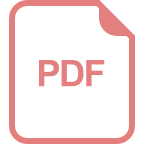




