用数据结构实现实现栈的应用表达式求值 采用顺序栈实现表达式求值 从运行界面输入一个表达式c 该表达式包括加法 减法 乘法 除法
时间: 2024-02-19 15:03:11 浏览: 27
好的,我会为您解答这个问题。
首先,我们需要将中缀表达式转换为后缀表达式,然后再通过后缀表达式求值。具体的步骤如下:
1. 定义操作符栈和操作数栈。
2. 从左到右扫描中缀表达式,如果是数字,则直接压入操作数栈;如果是操作符,则进行如下操作:
- 如果操作符栈为空或者栈顶操作符为左括号,则直接将该操作符压入操作符栈。
- 如果操作符为右括号,则将操作符栈中的操作符依次弹出并压入操作数栈,直到遇到左括号为止。
- 如果操作符的优先级大于栈顶操作符的优先级,则将该操作符压入操作符栈。
- 如果操作符的优先级小于等于栈顶操作符的优先级,则将栈顶操作符弹出并压入操作数栈,直到不再满足该条件为止,然后将该操作符压入操作符栈。
3. 如果中缀表达式已经扫描完毕,则将操作符栈中的所有操作符依次弹出并压入操作数栈。
4. 最后,操作数栈中的栈顶元素即为后缀表达式的值。
下面是用 C++ 实现的代码,其中假设操作符的优先级按照加减乘除从高到低依次为 3、2、1、0,左括号的优先级为 -1:
```c++
#include <iostream>
#include <stack>
#include <cctype>
using namespace std;
int precedence(char op) {
switch (op) {
case '+': case '-': return 2;
case '*': case '/': return 1;
case '(': return -1;
}
return -2; // 非法操作符
}
int evaluate(char op, int a, int b) {
switch (op) {
case '+': return a + b;
case '-': return a - b;
case '*': return a * b;
case '/': return a / b;
}
return 0; // 非法操作符
}
int evaluate_postfix(const string& expr) {
stack<int> operands;
for (char ch : expr) {
if (isdigit(ch)) {
operands.push(ch - '0');
} else if (ch == '+' || ch == '-' || ch == '*' || ch == '/') {
int b = operands.top(); operands.pop();
int a = operands.top(); operands.pop();
operands.push(evaluate(ch, a, b));
}
}
return operands.top();
}
int main() {
string expr;
cout << "请输入一个表达式:";
getline(cin, expr);
stack<char> operators;
string postfix;
for (char ch : expr) {
if (isdigit(ch)) {
postfix += ch;
} else if (ch == '+' || ch == '-' || ch == '*' || ch == '/') {
while (!operators.empty()
&& precedence(ch) <= precedence(operators.top())) {
postfix += operators.top();
operators.pop();
}
operators.push(ch);
} else if (ch == '(') {
operators.push(ch);
} else if (ch == ')') {
while (!operators.empty() && operators.top() != '(') {
postfix += operators.top();
operators.pop();
}
if (operators.empty()) {
cerr << "错误:缺少左括号!" << endl;
return 1;
}
operators.pop(); // 弹出左括号
} else if (!isspace(ch)) {
cerr << "错误:非法字符!" << endl;
return 1;
}
}
while (!operators.empty()) {
if (operators.top() == '(') {
cerr << "错误:缺少右括号!" << endl;
return 1;
}
postfix += operators.top();
operators.pop();
}
cout << "后缀表达式:" << postfix << endl;
cout << "计算结果:" << evaluate_postfix(postfix) << endl;
return 0;
}
```
希望这个回答能够帮到您!
相关推荐
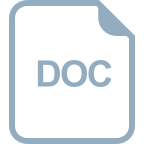
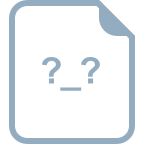
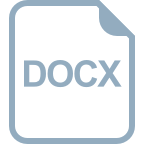














