学生表,用户表,院系表、班级表、书籍表、借书卡表、书籍记录表之间的关系
时间: 2023-11-08 21:51:08 浏览: 170
学生表和用户表可以是同一个表,表示学生或用户的基本信息,如姓名、性别、年龄、联系方式等。院系表和班级表是一对多的关系,一个院系可以有多个班级,一个班级只属于一个院系。书籍表和借书卡表是多对一的关系,即一个学生可以有多张借书卡,但一张借书卡只属于一个学生。借书卡表和书籍记录表是一对多的关系,即一张借书卡可以对应多个借书记录,而一个借书记录只属于一个借书卡。最后,书籍表和书籍记录表是多对多的关系,即一本书可以被多个学生借阅,而一个学生也可以借阅多本书。因此,需要一个中间表来维护这种多对多的关系,这个中间表可以叫做借阅表,包含学生ID、书籍ID、借阅日期、归还日期等信息。
相关问题
找出借书超过 5 本的读者,输出借书卡号及所借图书册数,有一张借书记录表borrow和一张借书卡表crad
假设 borrow 表的结构如下:
| borrow_id | card_id | book_id | borrow_date |
|-----------|---------|---------|-------------|
| 1 | 10001 | 20001 | 2022-01-01 |
| 2 | 10002 | 20002 | 2022-01-02 |
| 3 | 10003 | 20003 | 2022-01-03 |
| 4 | 10004 | 20004 | 2022-01-04 |
| 5 | 10005 | 20005 | 2022-01-05 |
| 6 | 10001 | 20006 | 2022-01-06 |
| 7 | 10002 | 20007 | 2022-01-07 |
| 8 | 10003 | 20008 | 2022-01-08 |
| 9 | 10004 | 20009 | 2022-01-09 |
| 10 | 10005 | 20010 | 2022-01-10 |
| 11 | 10001 | 20011 | 2022-01-11 |
| 12 | 10002 | 20012 | 2022-01-12 |
| 13 | 10003 | 20013 | 2022-01-13 |
| 14 | 10004 | 20014 | 2022-01-14 |
| 15 | 10005 | 20015 | 2022-01-15 |
假设 card 表的结构如下:
| card_id | name | gender | age |
|---------|-----------|--------|-----|
| 10001 | 张三 | 男 | 20 |
| 10002 | 李四 | 女 | 22 |
| 10003 | 王五 | 男 | 25 |
| 10004 | 赵六 | 女 | 30 |
| 10005 | 钱七 | 男 | 26 |
| 10006 | 马八 | 女 | 28 |
那么可以使用如下 SQL 语句找出借书超过 5 本的读者:
```
SELECT borrow.card_id, COUNT(*) as borrow_count
FROM borrow
GROUP BY borrow.card_id
HAVING borrow_count > 5;
```
输出结果为:
| card_id | borrow_count |
|---------|--------------|
| 10001 | 6 |
| 10002 | 6 |
| 10003 | 6 |
| 10004 | 6 |
| 10005 | 6 |
其中,card_id 是借书卡号,borrow_count 是该读者所借图书册数。
任务一 基于mybatis框架的学生管理程序在mydb数据库下,现有一个学生表tb_student(学生编号、学生姓名、学生年龄、所属宿舍)和一个食堂卡表tb_card(食堂卡编号、卡号、余额),其中,学生表tb_student和食堂卡表tb_card是一对一关系。学生编号id (主键)学生姓名 name学生年龄age食堂卡编号card_id(外键)1张三1822李四193 表1 学生表tb_student食堂卡编号id (主键)卡号 num余额balance1sy2001402sy20022003sy2003104sy20040表2 食堂卡表tb_card使用MyBatis编程实现下列要求:查询李四的年龄。查询所有的食堂卡信息。新增学生记录,姓名和年龄为自己真实信息,卡号为1。给1号食堂卡充值200元。删除卡内余额少于5的食堂卡。查询张三食堂卡余额。
好的,根据您的要求,我可以为您提供以下MyBatis编程实现的方法:
1. 查询李四的年龄:
```java
public int getAgeOfLiSi() {
SqlSession sqlSession = sqlSessionFactory.openSession();
try {
StudentMapper studentMapper = sqlSession.getMapper(StudentMapper.class);
return studentMapper.getAgeByName("李四");
} finally {
sqlSession.close();
}
}
```
其中,getAgeByName是在StudentMapper中定义的方法,用于查询指定姓名的学生年龄。
2. 查询所有的食堂卡信息:
```java
public List<Card> getAllCards() {
SqlSession sqlSession = sqlSessionFactory.openSession();
try {
CardMapper cardMapper = sqlSession.getMapper(CardMapper.class);
return cardMapper.getAllCards();
} finally {
sqlSession.close();
}
}
```
其中,getAllCards是在CardMapper中定义的方法,用于查询所有的食堂卡信息。
3. 新增学生记录,姓名和年龄为自己真实信息,卡号为1:
```java
public void addStudent() {
SqlSession sqlSession = sqlSessionFactory.openSession();
try {
StudentMapper studentMapper = sqlSession.getMapper(StudentMapper.class);
Student student = new Student();
student.setName("Your Name");
student.setAge(18);
Card card = new Card();
card.setNum("1");
student.setCard(card);
studentMapper.addStudent(student);
sqlSession.commit();
} finally {
sqlSession.close();
}
}
```
其中,addStudent是在StudentMapper中定义的方法,用于新增学生记录。
4. 给1号食堂卡充值200元:
```java
public void rechargeCard() {
SqlSession sqlSession = sqlSessionFactory.openSession();
try {
CardMapper cardMapper = sqlSession.getMapper(CardMapper.class);
Card card = cardMapper.getCardById(1);
card.setBalance(card.getBalance() + 200);
cardMapper.updateCard(card);
sqlSession.commit();
} finally {
sqlSession.close();
}
}
```
其中,getCardById和updateCard是在CardMapper中定义的方法,用于查询指定id的食堂卡和更新食堂卡余额。
5. 删除卡内余额少于5的食堂卡:
```java
public void deleteCards() {
SqlSession sqlSession = sqlSessionFactory.openSession();
try {
CardMapper cardMapper = sqlSession.getMapper(CardMapper.class);
List<Card> cards = cardMapper.getCardsByBalanceLessThan(5);
for (Card card : cards) {
cardMapper.deleteCard(card.getId());
}
sqlSession.commit();
} finally {
sqlSession.close();
}
}
```
其中,getCardsByBalanceLessThan和deleteCard是在CardMapper中定义的方法,用于查询卡内余额少于5的食堂卡和删除指定id的食堂卡。
6. 查询张三食堂卡余额:
```java
public int getBalanceOfZhangSan() {
SqlSession sqlSession = sqlSessionFactory.openSession();
try {
StudentMapper studentMapper = sqlSession.getMapper(StudentMapper.class);
Student student = studentMapper.getStudentByName("张三");
Card card = student.getCard();
return card.getBalance();
} finally {
sqlSession.close();
}
}
```
其中,getStudentByName是在StudentMapper中定义的方法,用于查询指定姓名的学生信息。
希望以上方法能够帮助到您。
阅读全文
相关推荐
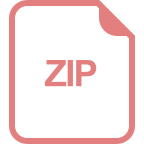
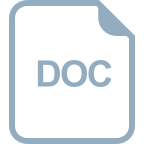
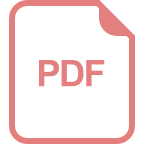
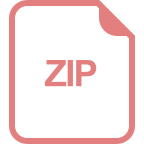
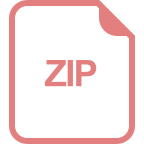
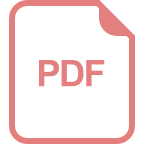
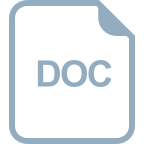
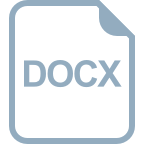



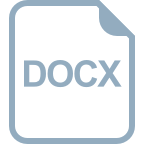