python 画个小猫咪
时间: 2023-11-12 19:59:56 浏览: 112
好的,我来给你展示一下如何用 Python 画一个小猫咪。
首先,我们需要导入 `turtle` 模块,这个模块可以让我们使用海龟绘图来画出图形。
```python
import turtle
```
然后,我们需要创建一个海龟对象,并设置一些属性,比如画笔颜色、画笔宽度等等。
```python
t = turtle.Turtle()
t.pensize(4)
t.pencolor('black')
```
接下来,我们就可以开始画小猫咪了。首先,我们画出小猫咪的头部和身体。
```python
# 画头部
t.circle(50)
# 画身体
t.penup()
t.goto(0, -70)
t.pendown()
t.circle(80)
```
然后,我们画出小猫咪的耳朵和眼睛。
```python
# 画左耳朵
t.penup()
t.goto(-30, 60)
t.pendown()
t.setheading(60)
t.circle(-30, 120)
# 画右耳朵
t.penup()
t.goto(30, 60)
t.pendown()
t.setheading(120)
t.circle(30, 120)
# 画左眼睛
t.penup()
t.goto(-20, 20)
t.pendown()
t.fillcolor('white')
t.begin_fill()
t.circle(15)
t.end_fill()
# 画右眼睛
t.penup()
t.goto(20, 20)
t.pendown()
t.fillcolor('white')
t.begin_fill()
t.circle(15)
t.end_fill()
# 画左瞳孔
t.penup()
t.goto(-20, 20)
t.pendown()
t.fillcolor('black')
t.begin_fill()
t.circle(8)
t.end_fill()
# 画右瞳孔
t.penup()
t.goto(20, 20)
t.pendown()
t.fillcolor('black')
t.begin_fill()
t.circle(8)
t.end_fill()
```
最后,我们画出小猫咪的鼻子和嘴巴。
```python
# 画鼻子
t.penup()
t.goto(0, 0)
t.pendown()
t.setheading(270)
t.fillcolor('pink')
t.begin_fill()
t.circle(20, -180)
t.end_fill()
# 画嘴巴
t.penup()
t.goto(0, -20)
t.pendown()
t.setheading(270)
t.circle(20, 180)
```
好了,现在我们已经成功地用 Python 画出了一个小猫咪。完整的代码如下:
```python
import turtle
# 创建海龟对象
t = turtle.Turtle()
# 设置画笔属性
t.pensize(4)
t.pencolor('black')
# 画头部
t.circle(50)
# 画身体
t.penup()
t.goto(0, -70)
t.pendown()
t.circle(80)
# 画左耳朵
t.penup()
t.goto(-30, 60)
t.pendown()
t.setheading(60)
t.circle(-30, 120)
# 画右耳朵
t.penup()
t.goto(30, 60)
t.pendown()
t.setheading(120)
t.circle(30, 120)
# 画左眼睛
t.penup()
t.goto(-20, 20)
t.pendown()
t.fillcolor('white')
t.begin_fill()
t.circle(15)
t.end_fill()
# 画右眼睛
t.penup()
t.goto(20, 20)
t.pendown()
t.fillcolor('white')
t.begin_fill()
t.circle(15)
t.end_fill()
# 画左瞳孔
t.penup()
t.goto(-20, 20)
t.pendown()
t.fillcolor('black')
t.begin_fill()
t.circle(8)
t.end_fill()
# 画右瞳孔
t.penup()
t.goto(20, 20)
t.pendown()
t.fillcolor('black')
t.begin_fill()
t.circle(8)
t.end_fill()
# 画鼻子
t.penup()
t.goto(0, 0)
t.pendown()
t.setheading(270)
t.fillcolor('pink')
t.begin_fill()
t.circle(20, -180)
t.end_fill()
# 画嘴巴
t.penup()
t.goto(0, -20)
t.pendown()
t.setheading(270)
t.circle(20, 180)
# 隐藏海龟
t.hideturtle()
# 点击关闭窗口
turtle.done()
```
相关推荐
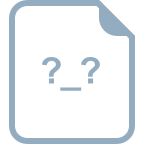
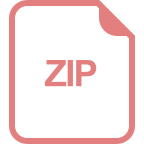














