编写Python代码检测工件缺陷并把缺陷画出来
时间: 2023-06-10 14:03:43 浏览: 103
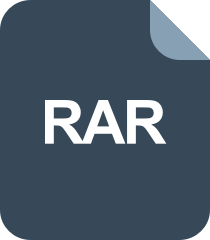
深度学习实现零件缺陷检测源代码

你可以使用OpenCV库来编写Python代码检测工件缺陷并把缺陷画出来。以下是大致思路:
1. 读取工件图片
2. 将图片转换为灰度图像
3. 使用Canny边缘检测算法检测边缘
4. 使用霍夫变换检测圆形缺陷或直线缺陷
5. 将检测出的边缘或缺陷用颜色标注在原图上
下面是示例代码:
```python
import cv2
import numpy as np
# 读取工件图片
img = cv2.imread('工件图片.jpg')
# 将图片转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 使用Canny边缘检测算法检测边缘
edges = cv2.Canny(gray, 50, 150, apertureSize=3)
# 使用霍夫变换检测圆形缺陷或直线缺陷
circles = cv2.HoughCircles(gray, cv2.HOUGH_GRADIENT, 1, 20,
param1=50, param2=30, minRadius=0, maxRadius=0)
lines = cv2.HoughLines(edges, 1, np.pi/180, 200)
# 将检测出的边缘或缺陷用颜色标注在原图上
if circles is not None:
circles = np.uint16(np.around(circles))
for i in circles[0,:]:
cv2.circle(img, (i[0], i[1]), i[2], (0, 255, 0), 2) # 画圆
else:
for rho, theta in lines[0]:
a = np.cos(theta)
b = np.sin(theta)
x0 = a*rho
y0 = b*rho
x1 = int(x0 + 1000*(-b))
y1 = int(y0 + 1000*(a))
x2 = int(x0 - 1000*(-b))
y2 = int(y0 - 1000*(a))
cv2.line(img, (x1, y1), (x2, y2), (0, 0, 255), 2) # 画线
cv2.imshow('defect detection', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请注意,以上仅为示例代码,具体实现可能需要根据实际情况进行修改。
阅读全文
相关推荐
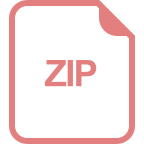
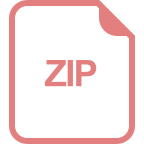












