给我来一个vue3的进销存软件代码
时间: 2024-01-26 14:04:08 浏览: 152
抱歉,我无法提供完整的进销存软件代码,因为这需要进行详细的需求分析和设计,然后编写大量的代码。不过,我可以提供一些Vue3的代码片段,可以帮助您开始构建进销存软件。
1. 安装Vue3和Vuex
首先,您需要安装Vue3和Vuex,可以使用npm或yarn进行安装。
```bash
# 使用npm安装
npm install vue@next vuex@next
# 使用yarn安装
yarn add vue@next vuex@next
```
2. 创建Vue3应用程序
您可以使用Vue CLI工具创建Vue3项目:
```bash
# 安装Vue CLI
npm install -g @vue/cli
# 创建Vue3项目
vue create my-app
```
3. 创建Vuex store
在Vuex store中,您可以存储应用程序的状态和实现业务逻辑。在store.js文件中,您可以创建Vuex store:
```javascript
import { createStore } from 'vuex'
const store = createStore({
state: {
products: [],
customers: [],
orders: [],
},
mutations: {
addProduct(state, product) {
state.products.push(product)
},
addCustomer(state, customer) {
state.customers.push(customer)
},
addOrder(state, order) {
state.orders.push(order)
},
},
actions: {
async fetchProducts(context) {
const response = await fetch('/api/products')
const products = await response.json()
context.commit('addProduct', products)
},
async fetchCustomers(context) {
const response = await fetch('/api/customers')
const customers = await response.json()
context.commit('addCustomer', customers)
},
async addOrder(context, order) {
await fetch('/api/orders', {
method: 'POST',
body: JSON.stringify(order),
})
context.commit('addOrder', order)
},
},
})
```
4. 创建Vue3组件
在Vue3组件中,您可以使用Vuex store中的数据和方法。例如,在ProductList.vue组件中,您可以使用fetchProducts方法从服务器获取产品列表,并在表格中显示它们:
```vue
<template>
<table>
<thead>
<tr>
<th>Name</th>
<th>Price</th>
<th>Stock</th>
</tr>
</thead>
<tbody>
<tr v-for="product in products" :key="product.id">
<td>{{ product.name }}</td>
<td>{{ product.price }}</td>
<td>{{ product.stock }}</td>
</tr>
</tbody>
</table>
</template>
<script>
import { computed } from 'vue'
import { useStore } from 'vuex'
export default {
setup() {
const store = useStore()
const products = computed(() => store.state.products)
store.dispatch('fetchProducts')
return { products }
},
}
</script>
```
这是一个简单的Vue3和Vuex的示例,您可以根据您的需求进行更改和扩展。但请记住,开发完整的进销存软件需要大量的工作和时间,并需要进行详细的需求分析和设计。
阅读全文
相关推荐
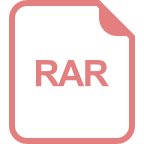
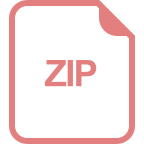
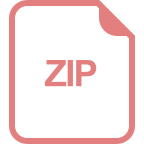
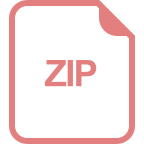
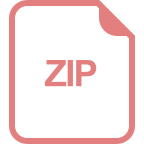
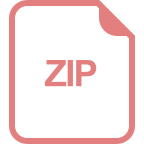
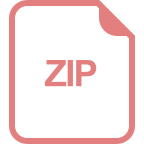
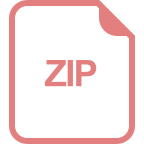
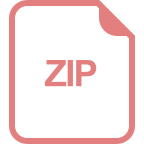
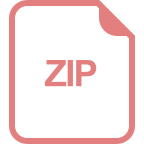
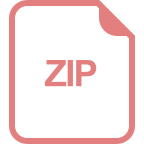
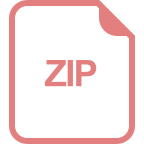
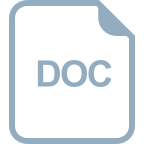
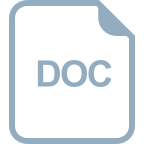
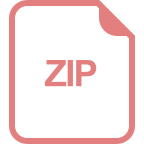