将强化学习策略梯度算法应用于迷宫问题
时间: 2023-07-26 18:39:53 浏览: 172
以下是一个简单的强化学习策略梯度算法应用于迷宫问题的Python代码示例,可以作为参考:
```python
import numpy as np
# 定义迷宫的大小和状态数
maze_size = (3, 4)
num_states = maze_size[0] * maze_size[1]
# 定义动作空间和动作数
action_space = [(0, 1), (0, -1), (1, 0), (-1, 0)]
num_actions = len(action_space)
# 定义策略网络
def init_policy_network():
policy_network = np.random.randn(num_states, num_actions)
return policy_network
# 定义策略函数
def policy_function(state):
action_probs = np.exp(policy_network[state]) / np.sum(np.exp(policy_network[state]))
return action_probs
# 定义奖励函数
def reward_function(state):
if state == num_states - 1:
return 1
else:
return 0
# 定义更新策略网络参数的函数
def update_policy_network(grad):
learning_rate = 0.1
policy_network += learning_rate * grad
# 开始训练
num_episodes = 1000
policy_network = init_policy_network()
for i in range(num_episodes):
# 初始化环境
state = 0
done = False
episode_rewards = []
# 采样一条轨迹
while not done:
# 选择动作
action_probs = policy_function(state)
action = np.random.choice(num_actions, p=action_probs)
action_delta = action_space[action]
# 执行动作,观察环境反馈
next_state = state + np.ravel_multi_index(action_delta, maze_size)
reward = reward_function(next_state)
episode_rewards.append(reward)
# 计算策略梯度
grad = np.zeros((num_states, num_actions))
grad[state, action] = 1 - action_probs[action]
for a in range(num_actions):
grad[state, a] -= action_probs[a] * sum([grad[next_state, a] for next_state in range(num_states)])
# 更新策略网络参数
update_policy_network(grad)
# 检查是否到达终点
if next_state == num_states - 1:
done = True
else:
state = next_state
# 打印每个回合的总回报
print(f"Episode {i+1}: total reward = {sum(episode_rewards)}")
```
在这个示例中,我们使用了一个简单的3x4迷宫环境,其中起点为状态0,终点为状态11。在每个回合中,我们通过采样随机动作来交互环境,并根据奖励函数计算每个状态的回报。我们使用策略梯度算法来更新策略网络参数,从而最大化总回报。
阅读全文
相关推荐
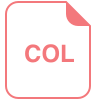
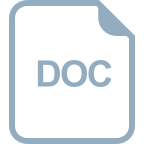
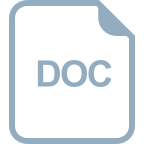
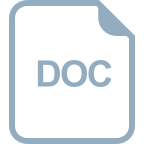
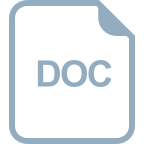
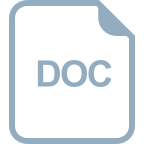
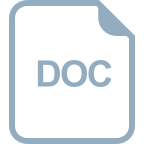
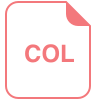
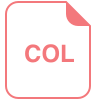
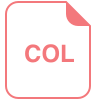
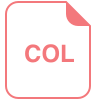
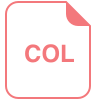
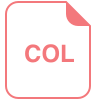
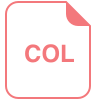
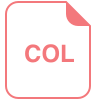
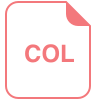