HTML搜索框输入城市名查询天气
时间: 2024-03-06 17:27:27 浏览: 55
这个问题需要使用 HTML、CSS、JavaScript 和天气 API 来实现。
首先,在 HTML 中创建一个搜索框和一个按钮:
```html
<input type="text" id="city" placeholder="输入城市名称">
<button onclick="searchWeather()">查询</button>
```
然后,在 JavaScript 中定义 `searchWeather` 函数,在函数中获取用户输入的城市名称,并将其作为参数发送到天气 API 请求天气数据:
```javascript
function searchWeather() {
var city = document.getElementById("city").value;
var url = "https://api.openweathermap.org/data/2.5/weather?q=" + city + "&appid=<your-api-key>";
// Send API request and handle response
// ...
}
```
在发送 API 请求前,需要在 [OpenWeatherMap](https://openweathermap.org/) 上注册账户并获取 API key,然后将其替换到 URL 中。
接着,使用 XMLHttpRequest 或 Fetch API 发送 API 请求,获取天气数据:
```javascript
function searchWeather() {
var city = document.getElementById("city").value;
var url = "https://api.openweathermap.org/data/2.5/weather?q=" + city + "&appid=<your-api-key>";
// Send API request and handle response
var xhr = new XMLHttpRequest();
xhr.open("GET", url);
xhr.onload = function() {
if (xhr.status === 200) {
var data = JSON.parse(xhr.responseText);
// Handle weather data
// ...
} else {
console.log("Request failed. Status: " + xhr.status);
}
};
xhr.send();
}
```
最后,将获取到的天气数据解析后显示在页面上:
```javascript
function searchWeather() {
var city = document.getElementById("city").value;
var url = "https://api.openweathermap.org/data/2.5/weather?q=" + city + "&appid=<your-api-key>";
// Send API request and handle response
var xhr = new XMLHttpRequest();
xhr.open("GET", url);
xhr.onload = function() {
if (xhr.status === 200) {
var data = JSON.parse(xhr.responseText);
// Handle weather data
var weather = data.weather[0].description;
var temp = (data.main.temp - 273.15).toFixed(1) + "°C";
var humidity = data.main.humidity + "%";
// Display weather data
var result = document.getElementById("result");
result.innerHTML = "<p>天气:" + weather + "</p><p>温度:" + temp + "</p><p>湿度:" + humidity + "</p>";
} else {
console.log("Request failed. Status: " + xhr.status);
}
};
xhr.send();
}
```
将结果显示在页面上的方式可以根据实际需求进行修改。完整的代码可以参考以下示例:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>查询天气</title>
</head>
<body>
<input type="text" id="city" placeholder="输入城市名称">
<button onclick="searchWeather()">查询</button>
<div id="result"></div>
<script>
function searchWeather() {
var city = document.getElementById("city").value;
var url = "https://api.openweathermap.org/data/2.5/weather?q=" + city + "&appid=<your-api-key>";
// Send API request and handle response
var xhr = new XMLHttpRequest();
xhr.open("GET", url);
xhr.onload = function() {
if (xhr.status === 200) {
var data = JSON.parse(xhr.responseText);
// Handle weather data
var weather = data.weather[0].description;
var temp = (data.main.temp - 273.15).toFixed(1) + "°C";
var humidity = data.main.humidity + "%";
// Display weather data
var result = document.getElementById("result");
result.innerHTML = "<p>天气:" + weather + "</p><p>温度:" + temp + "</p><p>湿度:" + humidity + "</p>";
} else {
console.log("Request failed. Status: " + xhr.status);
}
};
xhr.send();
}
</script>
</body>
</html>
```
阅读全文
相关推荐
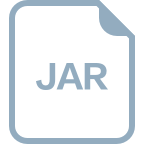














