操作系统设备管理c++代码实现_学长带你了解操作系统结构设计,设备管理
时间: 2023-12-20 16:07:35 浏览: 28
设备管理是操作系统中非常重要的一部分,其主要作用是为应用程序提供对硬件设备的访问。在这里我将为你介绍如何使用C++代码实现设备管理。
1. 设备驱动程序
设备驱动程序是操作系统与硬件之间的桥梁,其作用是将应用程序所发送的请求转化为硬件能够理解的指令并传递给硬件设备。其中,设备驱动程序的主要任务包括设备初始化、设备打开、设备关闭、设备读取和设备写入等。
以下是一个简单的设备驱动程序示例:
```c++
class DeviceDriver {
public:
virtual bool init() = 0;
virtual bool open() = 0;
virtual bool close() = 0;
virtual int read(void *buf, int count) = 0;
virtual int write(const void *buf, int count) = 0;
};
```
2. 设备管理器
设备管理器是操作系统中的一个组件,其主要作用是管理所有可用的硬件设备。在操作系统启动时,设备管理器将会扫描系统中所有的硬件设备,并将其信息存储在一个设备列表中。在应用程序需要访问某个硬件设备时,设备管理器将会根据设备的信息和应用程序的请求,选择合适的设备驱动程序,并将其加载到操作系统中。
以下是一个简单的设备管理器示例:
```c++
class DeviceManager {
public:
void scan_devices();
DeviceDriver* get_device_driver(const std::string& device_name);
private:
std::vector<DeviceDriver*> devices_;
};
```
3. 应用程序
应用程序是使用操作系统提供的设备管理功能访问硬件设备的主要途径。在应用程序中,我们可以使用设备管理器提供的接口来获取设备驱动程序,并通过设备驱动程序对硬件设备进行读写操作。
以下是一个简单的应用程序示例:
```c++
int main() {
DeviceManager dm;
dm.scan_devices();
DeviceDriver* device = dm.get_device_driver("serial_port_1");
device->open();
char buf[1024];
int count = device->read(buf, 1024);
// 处理读取到的数据
device->write("Hello, World!", 13);
// 发送数据到设备
device->close();
return 0;
}
```
以上就是使用C++代码实现设备管理的基本思路和示例。当然,实际应用中还需要考虑更多的细节和安全性问题。
相关推荐
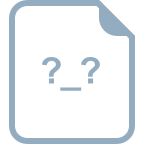
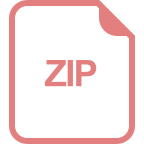
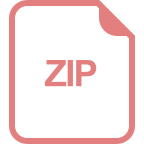














