51单片机串行口实现数据在lcd上显示代码
时间: 2024-03-14 10:44:17 浏览: 79
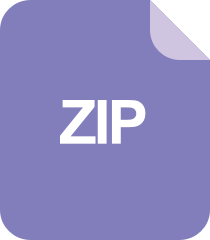
51单片机实现在lcd屏中显示键码值.zip
下面是一个基于51单片机的串口通信并将接收到的数据显示在LCD1602液晶屏上的代码:
```
#include <reg52.h>
#include <stdio.h>
#define LCD1602_RS P2^0 // LCD1602的RS引脚
#define LCD1602_RW P2^1 // LCD1602的RW引脚
#define LCD1602_EN P2^2 // LCD1602的EN引脚
#define LCD1602_DATAPINS P0 // LCD1602的数据引脚
sbit UART_RXD = P3^0; // 串口接收引脚
sbit UART_TXD = P3^1; // 串口发送引脚
void init_uart() // 初始化串口
{
TMOD = 0x20; // 设置定时器1为8位自动重载模式
TH1 = 0xfd; // 设置波特率为9600
TL1 = 0xfd;
TR1 = 1; // 启动定时器1
SCON = 0x50; // 设置串口为模式1,允许接收
ES = 1; // 允许串口中断
EA = 1; // 允许总中断
}
void init_lcd() // 初始化LCD
{
LCD1602_RS = 0;
LCD1602_RW = 0;
LCD1602_EN = 0;
delay_ms(15);
lcd_write_cmd(0x38); // 设置LCD为2行16列显示模式
delay_ms(5);
lcd_write_cmd(0x0c); // 开启LCD显示,光标不显示
delay_ms(5);
lcd_clear(); // 清屏
lcd_write_cmd(0x06); // 设置LCD写入数据后光标右移,不移动屏幕
}
void lcd_write_cmd(unsigned char cmd) // 写入LCD指令
{
LCD1602_RS = 0;
LCD1602_DATAPINS = cmd;
LCD1602_EN = 1;
delay_us(2);
LCD1602_EN = 0;
delay_ms(1);
}
void lcd_write_data(unsigned char dat) // 写入LCD数据
{
LCD1602_RS = 1;
LCD1602_DATAPINS = dat;
LCD1602_EN = 1;
delay_us(2);
LCD1602_EN = 0;
delay_ms(1);
}
void lcd_clear() // 清屏
{
lcd_write_cmd(0x01);
}
void lcd_set_cursor(unsigned char x, unsigned char y) // 设置光标位置
{
unsigned char addr;
if (y == 0)
addr = 0x80 + x;
else
addr = 0xc0 + x;
lcd_write_cmd(addr);
}
void lcd_puts(unsigned char x, unsigned char y, unsigned char *str) // 输出字符串到LCD
{
lcd_set_cursor(x, y);
while (*str != '\0')
{
lcd_write_data(*str);
str++;
}
}
void uart_isr() interrupt 4 // 串口中断处理函数
{
if (RI == 1) // 如果接收到数据
{
RI = 0; // 清除接收标志位
lcd_write_data(SBUF); // 将接收到的数据写入LCD
}
}
void main()
{
init_uart(); // 初始化串口
init_lcd(); // 初始化LCD
while (1); // 主循环
}
```
在这段代码中,我们定义了LCD1602的控制引脚和数据引脚,以及串口的接收引脚和发送引脚。在初始化函数中,我们通过调用lcd_write_cmd()函数向LCD发送指令,设置LCD为2行16列显示模式,并开启显示。在uart_isr()函数中,我们通过lcd_write_data()函数将接收到的数据写入LCD。在主函数中,我们调用了init_uart()和init_lcd()函数进行初始化,并进入了一个死循环中。
阅读全文
相关推荐

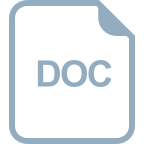

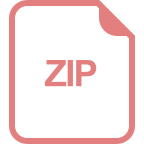
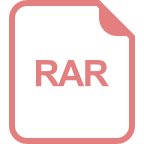
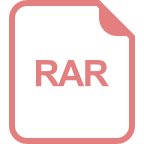
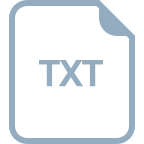
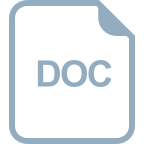
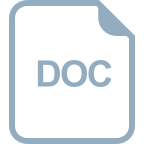
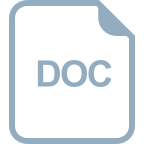
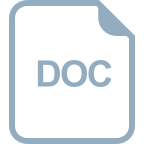
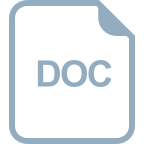
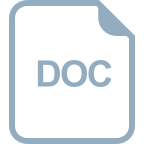
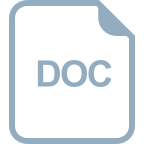
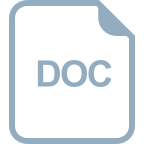