编写一个C语言程序,给定一个整数数组 nums,将所有 0 移动到数组的末尾,同时保持非零元素的相对顺序。
时间: 2024-02-09 09:10:35 浏览: 76
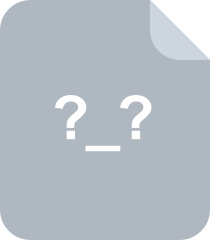
python 移动零,给定一个数组 nums,编写一个函数将所有 0 移动到数组的末尾,同时保持非零元素的相对顺序

好的,下面是一个简单的 C 语言程序,可以实现你的要求:
```c
#include <stdio.h>
void moveZeroes(int* nums, int numsSize) {
int i, j = 0;
for (i = 0; i < numsSize; i++) {
if (nums[i] != 0) {
nums[j++] = nums[i];
}
}
for (i = j; i < numsSize; i++) {
nums[i] = 0;
}
}
int main() {
int nums[] = {0, 1, 0, 3, 12};
int numsSize = sizeof(nums) / sizeof(nums[0]);
moveZeroes(nums, numsSize);
for (int i = 0; i < numsSize; i++) {
printf("%d ", nums[i]);
}
return 0;
}
```
这个程序中,我们定义了一个函数 `moveZeroes`,它接受一个整数数组和数组的大小作为参数。该函数的作用是将数组中的所有 0 移动到数组的末尾,同时保持非零元素的相对顺序。具体实现方式是,使用两个指针 i 和 j,i 从头开始遍历数组,j 记录下一个非零元素应该放置的位置,当遇到非零元素时,将该元素赋值给 nums[j],并将 j 加 1;最后,将 nums[j] 到 nums[numsSize-1] 的元素全部置为 0。
在主函数中,我们定义了一个示例数组 nums,调用了 `moveZeroes` 函数,然后输出结果。运行程序,输出的结果应该是:
```
1 3 12 0 0
```
希望这个程序能够帮到你!
阅读全文
相关推荐















