hugelnteger类c++
时间: 2024-05-06 12:16:08 浏览: 209
HugeInteger是一个自定义的C++类,用于表示和操作大整数(超过了标准数据类型的范围)。下面是一个简单的实现,可以进行加法、减法、乘法和比较操作:
```c++
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
class HugeInteger {
private:
static const int MAX_SIZE = 1000;
int digits[MAX_SIZE];
bool isNegative;
int size;
public:
HugeInteger() {
fill(digits, digits + MAX_SIZE, 0);
isNegative = false;
size = 0;
}
HugeInteger(string s) {
fill(digits, digits + MAX_SIZE, 0);
isNegative = false;
size = 0;
// 处理符号位
if (s[0] == '-') {
isNegative = true;
s.erase(0, 1);
}
// 处理前导零
while (s.size() > 1 && s[0] == '0') {
s.erase(0, 1);
}
// 将字符串转换为数字数组
for (int i = s.size() - 1, j = 0; i >= 0; i--, j++) {
digits[j] = s[i] - '0';
size++;
}
}
string toString() {
string s = "";
// 处理符号位
if (isNegative) {
s += "-";
}
// 转换数字数组为字符串
for (int i = size - 1; i >= 0; i--) {
s += to_string(digits[i]);
}
return s;
}
void trim() {
// 去掉前导零
while (size > 1 && digits[size - 1] == 0) {
size--;
}
// 判断结果是否为0
if (size == 1 && digits[0] == 0) {
isNegative = false;
}
}
HugeInteger operator+(HugeInteger b) {
HugeInteger result;
// 判断符号,转换为加法或减法
if (isNegative == b.isNegative) {
int carry = 0;
for (int i = 0; i < max(size, b.size) || carry; i++) {
int sum = digits[i] + b.digits[i] + carry;
result.digits[result.size++] = sum % 10;
carry = sum / 10;
}
result.isNegative = isNegative;
} else {
// 符号不同,转换为减法
HugeInteger absA = *this;
HugeInteger absB = b;
absA.isNegative = false;
absB.isNegative = false;
if (absA < absB) {
result = absB - absA;
result.isNegative = b.isNegative;
} else {
int borrow = 0;
for (int i = 0; i < max(size, b.size); i++) {
int diff = digits[i] - b.digits[i] - borrow;
if (diff < 0) {
diff += 10;
borrow = 1;
} else {
borrow = 0;
}
result.digits[result.size++] = diff;
}
result.isNegative = isNegative;
}
}
result.trim();
return result;
}
HugeInteger operator-(HugeInteger b) {
HugeInteger result;
// 判断符号,转换为加法或减法
if (isNegative == b.isNegative) {
HugeInteger absA = *this;
HugeInteger absB = b;
absA.isNegative = false;
absB.isNegative = false;
if (absA < absB) {
result = absB - absA;
result.isNegative = !isNegative;
} else {
int borrow = 0;
for (int i = 0; i < max(size, b.size); i++) {
int diff = digits[i] - b.digits[i] - borrow;
if (diff < 0) {
diff += 10;
borrow = 1;
} else {
borrow = 0;
}
result.digits[result.size++] = diff;
}
result.isNegative = isNegative;
}
} else {
// 符号不同,转换为加法
HugeInteger absA = *this;
HugeInteger absB = b;
absA.isNegative = false;
absB.isNegative = false;
result = absA + absB;
result.isNegative = isNegative;
}
result.trim();
return result;
}
HugeInteger operator*(HugeInteger b) {
HugeInteger result;
for (int i = 0; i < size; i++) {
int carry = 0;
for (int j = 0; j < b.size || carry; j++) {
int product = digits[i] * b.digits[j] + carry + result.digits[i + j];
result.digits[i + j] = product % 10;
carry = product / 10;
}
}
result.size = size + b.size - 1;
result.isNegative = isNegative ^ b.isNegative;
result.trim();
return result;
}
bool operator<(HugeInteger b) {
if (isNegative != b.isNegative) {
return isNegative;
}
if (size != b.size) {
return (size < b.size) ^ isNegative;
}
for (int i = size - 1; i >= 0; i--) {
if (digits[i] != b.digits[i]) {
return (digits[i] < b.digits[i]) ^ isNegative;
}
}
return false;
}
bool operator>(HugeInteger b) {
return b < *this;
}
bool operator<=(HugeInteger b) {
return !(b < *this);
}
bool operator>=(HugeInteger b) {
return !(*this < b);
}
bool operator==(HugeInteger b) {
return !(*this < b || b < *this);
}
bool operator!=(HugeInteger b) {
return (*this < b || b < *this);
}
};
int main() {
HugeInteger a("123456789012345678901234567890");
HugeInteger b("-987654321098765432109876543210");
HugeInteger c = a + b;
HugeInteger d = a - b;
HugeInteger e = a * b;
cout << "a = " << a.toString() << endl;
cout << "b = " << b.toString() << endl;
cout << "a + b = " << c.toString() << endl;
cout << "a - b = " << d.toString() << endl;
cout << "a * b = " << e.toString() << endl;
if (a < b) {
cout << "a < b" << endl;
}
if (a > b) {
cout << "a > b" << endl;
}
if (a <= b) {
cout << "a <= b" << endl;
}
if (a >= b) {
cout << "a >= b" << endl;
}
if (a == b) {
cout << "a == b" << endl;
}
if (a != b) {
cout << "a != b" << endl;
}
return 0;
}
```
这个实现还不完善,只支持非负整数,没有处理除法和取模运算。如果需要更完整的实现,可以参考一些第三方库,如GMP和NTL等。
阅读全文
相关推荐
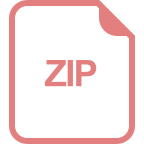
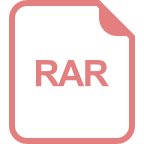
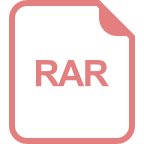
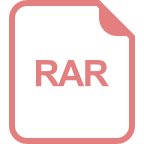
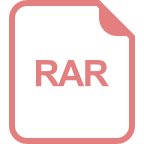
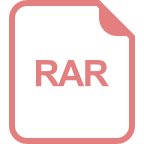