用Java 写一段转账的代码
时间: 2024-03-16 22:42:25 浏览: 50
好的,以下是一个基本的 Java 转账代码示例:
```java
import java.util.Scanner;
public class TransferMoney {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入转出账户名:");
String fromAccount = scanner.nextLine();
System.out.println("请输入转入账户名:");
String toAccount = scanner.nextLine();
System.out.println("请输入转账金额:");
double amount = scanner.nextDouble();
scanner.close();
// 模拟转账操作
boolean success = transfer(fromAccount, toAccount, amount);
if (success) {
System.out.println("转账成功!");
} else {
System.out.println("转账失败,请检查账户余额!");
}
}
/**
* 转账操作
* @param fromAccount 转出账户
* @param toAccount 转入账户
* @param amount 转账金额
* @return 转账是否成功
*/
public static boolean transfer(String fromAccount, String toAccount, double amount) {
// 模拟账户余额
double balance = 1000.0;
// 判断账户余额是否足够
if (balance >= amount) {
balance -= amount; // 转出账户减去转账金额
System.out.println(fromAccount + " 转出 " + amount + " 元,账户余额为 " + balance + " 元。");
System.out.println(toAccount + " 收到 " + amount + " 元。");
return true;
} else {
return false;
}
}
}
```
这个程序需要用户输入转出账户名、转入账户名和转账金额,然后调用 `transfer` 方法模拟转账操作。在 `transfer` 方法中,首先模拟了账户余额为 1000 元,然后判断账户余额是否足够执行转账操作。如果账户余额足够,就执行转账操作并返回 true,否则返回 false。最后根据 `transfer` 方法的返回值判断转账是否成功,并输出相应的结果。
阅读全文
相关推荐

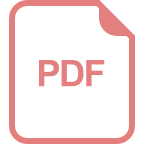
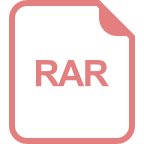
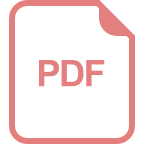
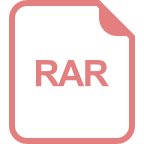
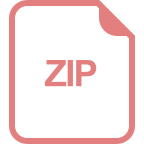
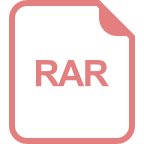
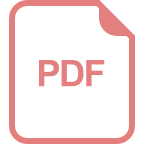
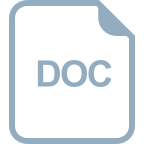
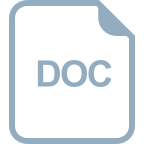
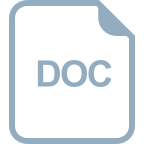
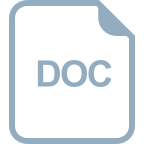
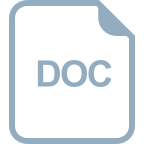
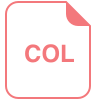
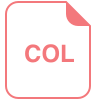
