用python写一个熊猫坐瓢虫的游戏
时间: 2024-05-10 08:18:33 浏览: 10
好的,下面是一个简单的熊猫坐瓢虫游戏代码示例,需要用到Python的pygame库:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置屏幕大小和标题
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("Panda and Ladybug")
# 加载图片
panda_img = pygame.image.load("panda.png").convert_alpha()
ladybug_img = pygame.image.load("ladybug.png").convert_alpha()
# 设置游戏参数
panda_pos = [screen_width // 2, screen_height // 2] # 熊猫初始位置
ladybug_list = [] # 瓢虫列表
score = 0
# 创建瓢虫类
class Ladybug:
def __init__(self):
self.x = random.randint(0, screen_width - 50)
self.y = random.randint(0, screen_height - 50)
self.speed = 2
def move(self):
direction = random.choice(["up", "down", "left", "right"])
if direction == "up":
self.y -= self.speed
elif direction == "down":
self.y += self.speed
elif direction == "left":
self.x -= self.speed
elif direction == "right":
self.x += self.speed
# 检查瓢虫是否出界
if self.x < 0:
self.x = 0
elif self.x > screen_width - 50:
self.x = screen_width - 50
if self.y < 0:
self.y = 0
elif self.y > screen_height - 50:
self.y = screen_height - 50
def draw(self):
screen.blit(ladybug_img, (self.x, self.y))
# 创建多个瓢虫
for i in range(10):
ladybug = Ladybug()
ladybug_list.append(ladybug)
# 游戏循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 移动瓢虫
for ladybug in ladybug_list:
ladybug.move()
# 检查熊猫是否碰到瓢虫
for ladybug in ladybug_list:
if (panda_pos[0] < ladybug.x + 50 and panda_pos[0] + 50 > ladybug.x and
panda_pos[1] < ladybug.y + 50 and panda_pos[1] + 50 > ladybug.y):
score += 1
ladybug_list.remove(ladybug)
# 移动熊猫
keys = pygame.key.get_pressed()
if keys[pygame.K_UP]:
panda_pos[1] -= 5
elif keys[pygame.K_DOWN]:
panda_pos[1] += 5
elif keys[pygame.K_LEFT]:
panda_pos[0] -= 5
elif keys[pygame.K_RIGHT]:
panda_pos[0] += 5
# 检查熊猫是否出界
if panda_pos[0] < 0:
panda_pos[0] = 0
elif panda_pos[0] > screen_width - 50:
panda_pos[0] = screen_width - 50
if panda_pos[1] < 0:
panda_pos[1] = 0
elif panda_pos[1] > screen_height - 50:
panda_pos[1] = screen_height - 50
# 绘制游戏场景
screen.fill((255, 255, 255))
screen.blit(panda_img, tuple(panda_pos))
for ladybug in ladybug_list:
ladybug.draw()
# 绘制分数
font = pygame.font.Font(None, 36)
score_text = font.render("Score: {}".format(score), True, (0, 0, 0))
screen.blit(score_text, (10, 10))
# 更新屏幕
pygame.display.update()
# 退出Pygame
pygame.quit()
```
在代码中,我们创建了一个Ladybug类表示瓢虫,它包含瓢虫的位置和移动方法。我们创建了多个瓢虫,并在游戏循环中移动它们。我们还检查熊猫是否碰到了瓢虫,并根据情况增加得分。最后我们在屏幕上绘制了游戏场景和分数,并更新屏幕。
相关推荐
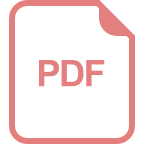
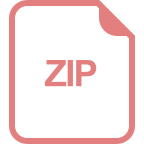
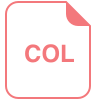
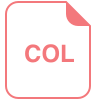
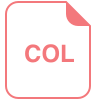
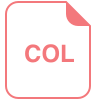
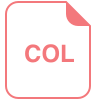









