web.py 详细教程
时间: 2023-07-28 10:07:18 浏览: 120
web.py 是一个轻量级的 Python web 框架,它提供了一些简单易用的工具和 API,帮助你快速构建 web 应用程序。下面是 web.py 的详细教程:
1. 安装 web.py
你可以使用 pip 安装 web.py:
```
pip install web.py
```
2. 创建一个 web 应用程序
创建一个 Python 文件,比如 `app.py`,并写入以下代码:
```python
import web
urls = (
'/', 'index'
)
class index:
def GET(self):
return "Hello, world!"
if __name__ == "__main__":
app = web.application(urls, globals())
app.run()
```
这个应用程序只有一个路由,当访问根路径时,会返回字符串 `"Hello, world!"`。
3. 运行应用程序
在终端中运行 `python app.py`,你会看到以下输出:
```
http://0.0.0.0:8080/
```
在浏览器中访问 `http://localhost:8080/`,你应该能够看到字符串 `"Hello, world!"`。
4. 添加更多路由
你可以通过在 `urls` 元组中添加更多路由来扩展应用程序:
```python
import web
urls = (
'/', 'index',
'/hello', 'hello'
)
class index:
def GET(self):
return "Hello, world!"
class hello:
def GET(self):
return "Hello, there!"
if __name__ == "__main__":
app = web.application(urls, globals())
app.run()
```
现在,当访问根路径时,会返回字符串 `"Hello, world!"`,当访问 `/hello` 路径时,会返回字符串 `"Hello, there!"`。
5. 接收参数
你可以在 URL 中使用占位符来接收参数:
```python
import web
urls = (
'/hello/(.*)', 'hello'
)
class hello:
def GET(self, name):
return "Hello, " + name + "!"
if __name__ == "__main__":
app = web.application(urls, globals())
app.run()
```
现在当你访问 `/hello/world` 路径时,会返回字符串 `"Hello, world!"`。
6. 使用模板
你可以使用 web.py 内置的模板引擎来渲染 HTML 页面:
```python
import web
render = web.template.render('templates/')
urls = (
'/', 'index'
)
class index:
def GET(self):
return render.index()
if __name__ == "__main__":
app = web.application(urls, globals())
app.run()
```
在 `templates/` 目录下创建一个名为 `index.html` 的文件,写入以下代码:
```html
<!doctype html>
<html>
<head>
<title>Hello, world!</title>
</head>
<body>
<h1>Hello, world!</h1>
</body>
</html>
```
现在当你访问根路径时,会返回渲染后的 HTML 页面。
这就是 web.py 的基本用法,你可以在官方文档中了解更多。
阅读全文
相关推荐
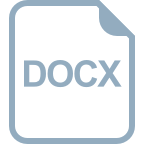
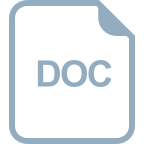
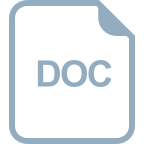
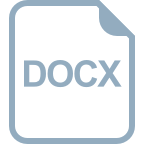
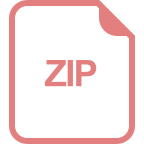
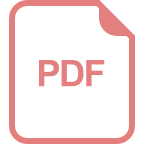
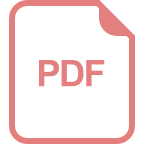
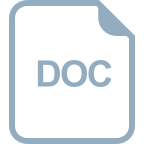
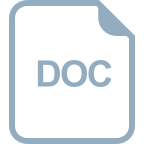
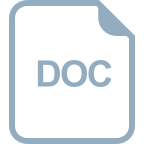
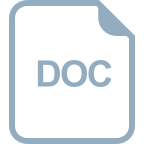
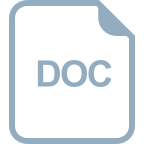
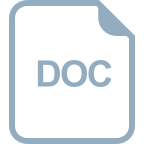
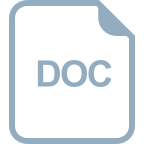
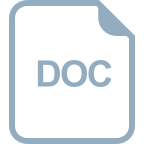