自定义完成图形颜色和面积的计算,矩形和三角形的面积c++
时间: 2024-09-28 09:05:30 浏览: 31
在C++中,自定义完成图形颜色和面积的计算,特别是对于矩形和三角形,你可以通过创建类来封装几何形状的基本属性(如颜色和边长)以及它们的面积计算方法。下面是一个简单的例子:
首先,创建两个类:Rectangle 和 Triangle,分别代表矩形和三角形。
```cpp
#include <iostream>
#include <string>
// 定义矩形类
class Rectangle {
private:
std::string color;
double width, height;
public:
Rectangle(const std::string& c, double w, double h) : color(c), width(w), height(h) {}
// 面积计算方法
double getArea() const { return width * height; }
void setColor(const std::string& newColor) { color = newColor; } // 设置颜色
std::string getColor() const { return color; } // 获取颜色
};
// 定义三角形类
class Triangle {
private:
std::string color;
double base, height;
public:
Triangle(const std::string& c, double b, double h) : color(c), base(b), height(h) {}
// 面积计算方法,海伦公式
double getArea() const {
double s = (base + height + sqrt(base * base + height * height)) / 2.0;
return sqrt(s * (s - base) * (s - height) * (s - base - height));
}
void setColor(const std::string& newColor) { color = newColor; } // 设置颜色
std::string getColor() const { return color; } // 获取颜色
};
```
现在你可以实例化这两个类并操作它们的颜色和面积:
```cpp
int main() {
Rectangle rect("blue", 5, 4);
rect.setColor("red");
std::cout << "Rectangle area: " << rect.getArea() << std::endl;
Triangle triangle("green", 3, 6);
triangle.setColor("yellow");
std::cout << "Triangle area: " << triangle.getArea() << std::endl;
return 0;
}
```
阅读全文
相关推荐
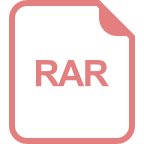
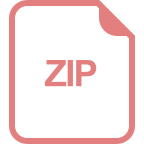
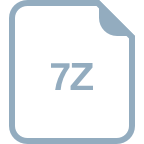
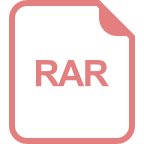
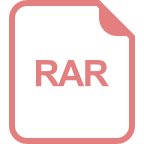
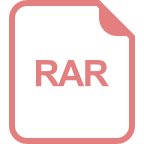
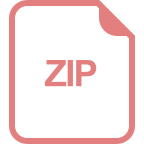
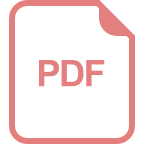
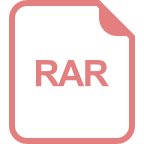
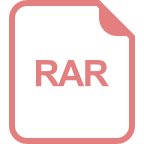
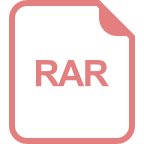
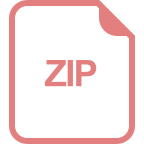
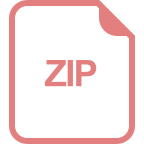
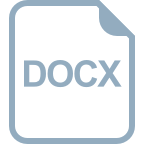
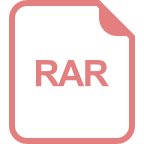
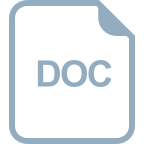
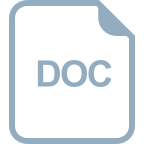
