一个电表按照如下的比率计费:前200度电:每度0.8元:后100度电:每度0.9元:超过300度电:每度1元。所有用户都是按最少100元进行收费。如果总费 用大于400元,还要加收总数的15%的费用。请编写程序,从键盘输入用电量,经过计算后输出显示应收电费,c语言
时间: 2024-10-16 08:22:01 浏览: 50
在C语言中,你可以创建一个函数来计算用户的电费。首先,我们需要读取用户输入的电量,然后根据不同的电价段计算费用,并考虑附加费用。下面是一个简单的示例代码:
```c
#include <stdio.h>
// 定义价格函数
float calculate_fee(int total_kwh) {
float base_fee = 0;
// 计算前200度电费用
if (total_kwh <= 200) {
base_fee = total_kwh * 0.8;
} else {
// 分段计算
base_fee += 200 * 0.8; // 前200度费用
int extra_kwh = total_kwh - 200; // 超过部分
// 后100度电费用
if (extra_kwh <= 100) {
base_fee += extra_kwh * 0.9;
} else {
// 超过300度费用
base_fee += 100 * 0.9; // 后100度费用
extra_kwh -= 100;
base_fee += extra_kwh * 1.0; // 超过300度的费用
}
}
// 检查是否需要加收15%附加费
if (base_fee > 400) {
base_fee *= 1.15; // 加上15%的附加费
}
return base_fee;
}
int main() {
int total_kwh;
printf("请输入您的用电量(整数): ");
scanf("%d", &total_kwh);
float fee = calculate_fee(total_kwh);
printf("您的电费总额是: %.2f 元\n", fee);
return 0;
}
```
这个程序会提示用户输入用电量,然后调用`calculate_fee`函数计算并打印出费用。注意,这里假设用户输入的是整数。
阅读全文
相关推荐
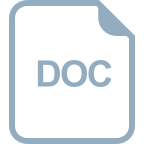
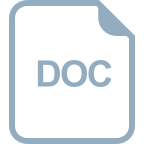
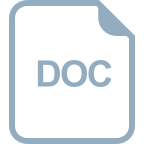







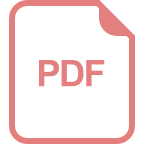
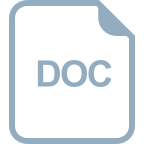
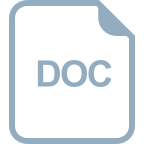



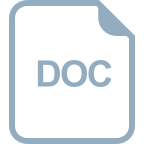