深度强化学习路径规划c++代码
时间: 2023-09-02 18:07:10 浏览: 50
深度强化学习路径规划在 C++ 中的实现可以使用 TensorFlow 等深度学习框架。以下是一个简单的 C++ 代码示例,演示了如何使用 TensorFlow 来实现深度强化学习路径规划:
```c++
#include <iostream>
#include <tensorflow/core/public/session.h>
#include <tensorflow/core/platform/env.h>
using namespace std;
using namespace tensorflow;
int main() {
// 创建 TensorFlow 会话
Session* session;
Status status = NewSession(SessionOptions(), &session);
if (!status.ok()) {
cout << "创建 TensorFlow 会话失败: " << status.ToString() << endl;
return 1;
}
// 加载模型
GraphDef graph_def;
status = ReadBinaryProto(Env::Default(), "my_model.pb", &graph_def);
if (!status.ok()) {
cout << "加载模型失败: " << status.ToString() << endl;
return 1;
}
status = session->Create(graph_def);
if (!status.ok()) {
cout << "创建图表失败: " << status.ToString() << endl;
return 1;
}
// 准备输入数据
Tensor input(DT_FLOAT, TensorShape({1, 10}));
auto input_map = input.tensor<float, 2>();
for (int i = 0; i < 10; ++i) {
input_map(0, i) = i;
}
// 运行模型
vector<pair<string, Tensor>> inputs = {{"input", input}};
vector<string> output_names = {"output"};
vector<Tensor> outputs;
status = session->Run(inputs, output_names, {}, &outputs);
if (!status.ok()) {
cout << "运行模型失败: " << status.ToString() << endl;
return 1;
}
// 输出结果
auto output_map = outputs[0].tensor<float, 2>();
for (int i = 0; i < 1; ++i) {
for (int j = 0; j < 10; ++j) {
cout << output_map(i, j) << endl;
}
}
// 释放资源
session->Close();
return 0;
}
```
在上面的示例中,我们首先创建了 TensorFlow 会话,然后加载了一个模型。接下来,我们准备输入数据并运行模型,最后输出结果并释放资源。请注意,这个示例中的模型是一个简单的前向神经网络,输入是一个形状为 (1, 10) 的张量,输出也是一个形状为 (1, 10) 的张量。在实际应用中,您需要根据具体情况修改代码。
相关推荐
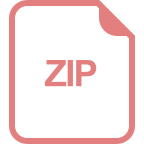














