二叉树基本操作演示程序c语言实现
时间: 2023-10-08 10:09:01 浏览: 51
以下是一个简单的二叉树基本操作演示程序的C语言实现:
```c
#include<stdio.h>
#include<stdlib.h>
struct node
{
int data;
struct node *left;
struct node *right;
};
struct node* create_node(int value)
{
struct node *temp = (struct node*)malloc(sizeof(struct node));
temp->data = value;
temp->left = NULL;
temp->right = NULL;
return temp;
}
struct node* insert(struct node *root, int value)
{
if(root == NULL)
{
return create_node(value);
}
else if(value <= root->data)
{
root->left = insert(root->left, value);
}
else
{
root->right = insert(root->right, value);
}
return root;
}
void inorder_traversal(struct node *root)
{
if(root == NULL)
{
return;
}
inorder_traversal(root->left);
printf("%d ", root->data);
inorder_traversal(root->right);
}
void preorder_traversal(struct node *root)
{
if(root == NULL)
{
return;
}
printf("%d ", root->data);
preorder_traversal(root->left);
preorder_traversal(root->right);
}
void postorder_traversal(struct node *root)
{
if(root == NULL)
{
return;
}
postorder_traversal(root->left);
postorder_traversal(root->right);
printf("%d ", root->data);
}
int main()
{
struct node *root = NULL;
root = insert(root, 5);
root = insert(root, 3);
root = insert(root, 7);
root = insert(root, 1);
root = insert(root, 9);
printf("Inorder Traversal: ");
inorder_traversal(root);
printf("\n");
printf("Preorder Traversal: ");
preorder_traversal(root);
printf("\n");
printf("Postorder Traversal: ");
postorder_traversal(root);
printf("\n");
return 0;
}
```
该程序定义了一个结构体 `node`,其中包含了一个整数值 `data`、一个指向左子树的指针 `left` 和一个指向右子树的指针 `right`。`create_node` 函数用于创建一个新的节点,并将传入的值存储在该节点的 `data` 中。`insert` 函数用于插入一个新的节点到二叉树中,若二叉树为空则直接新建一个节点,否则按照二叉搜索树的规则插入到左子树或右子树中。`inorder_traversal`、`preorder_traversal` 和 `postorder_traversal` 函数分别实现了中序遍历、前序遍历和后序遍历的功能。
在 `main` 函数中,首先创建了一个空的二叉树 `root`,然后插入了一些节点,最后对该二叉树进行了中序遍历、前序遍历和后序遍历,并输出结果。
相关推荐
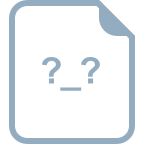
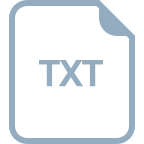
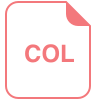








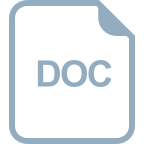
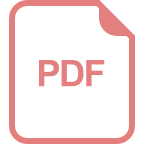
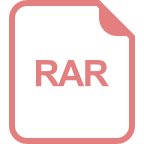
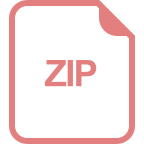