幫我寫一個基於pytorch環境,有使用pretrain,two stream early fusion的架構,包含載入rgb圖片與光流圖片路徑
时间: 2024-02-15 19:01:43 浏览: 65
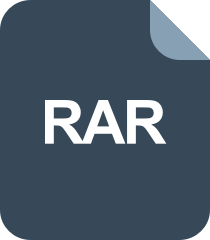
基于pytorch实现的、带GUI的图像风格迁移程序.
好的,以下是一个基于PyTorch环境,使用pretrain的Two Stream Early Fusion网络,并包含载入RGB图像和光流图像路径的代码:
```python
import torch
import torch.nn as nn
import torchvision.models as models
import cv2
import numpy as np
class TwoStreamEarlyFusion(nn.Module):
def __init__(self, num_classes):
super(TwoStreamEarlyFusion, self).__init__()
self.rgb_vgg16 = models.vgg16(pretrained=True)
self.opt_vgg16 = models.vgg16(pretrained=True)
self.rgb_fc = nn.Linear(4096, num_classes)
self.opt_fc = nn.Linear(4096, num_classes)
self.fusion_fc = nn.Linear(num_classes * 2, num_classes)
def forward(self, rgb, opt):
rgb_feats = self.rgb_vgg16.features(rgb)
rgb_feats = self.rgb_vgg16.avgpool(rgb_feats)
rgb_feats = torch.flatten(rgb_feats, 1)
rgb_feats = self.rgb_vgg16.classifier(rgb_feats)
rgb_feats = self.rgb_fc(rgb_feats)
opt_feats = self.opt_vgg16.features(opt)
opt_feats = self.opt_vgg16.avgpool(opt_feats)
opt_feats = torch.flatten(opt_feats, 1)
opt_feats = self.opt_vgg16.classifier(opt_feats)
opt_feats = self.opt_fc(opt_feats)
fusion_feats = torch.cat([rgb_feats, opt_feats], dim=1)
output = self.fusion_fc(fusion_feats)
return output
def load_rgb_image(filename):
img = cv2.imread(filename)
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
img = cv2.resize(img, (224, 224))
img = img.astype(np.float32) / 255.0
img = (img - np.array([0.485, 0.456, 0.406])) / np.array([0.229, 0.224, 0.225])
img = np.transpose(img, (2, 0, 1))
img = np.expand_dims(img, axis=0)
img = torch.from_numpy(img)
return img
def load_optical_flow(filename):
flow = cv2.imread(filename, cv2.IMREAD_GRAYSCALE)
flow = cv2.resize(flow, (224, 224))
flow = flow.astype(np.float32) / 255.0
flow = (flow - 0.5) / 0.226
flow = np.expand_dims(flow, axis=0)
flow = np.expand_dims(flow, axis=0)
flow = torch.from_numpy(flow)
return flow
```
这个架构的基本思想是:将RGB图像和光流图像分别输入两个VGG16网络中,然后将RGB网络和光流网络提取出来的特征拼接起来,再通过一个全连接层进行融合,最终输出分类结果。在这个架构中,我们使用了预训练的VGG16网络,并且定义了两个全连接层(rgb_fc和opt_fc)和一个融合层(fusion_fc)。
下面是载入RGB图像和光流图像路径的代码:
```python
rgb_path = 'path/to/rgb/image.jpg'
opt_path = 'path/to/optical/flow/image.jpg'
rgb_image = load_rgb_image(rgb_path)
opt_image = load_optical_flow(opt_path)
model = TwoStreamEarlyFusion(num_classes=10)
output = model(rgb_image, opt_image)
```
这里我们使用了OpenCV库来读取图像,并将RGB图像和光流图像都resize到224x224的大小。对于RGB图像,我们对其进行了标准化处理,使其像素值的范围在[0, 1]之间,并进行了零均值归一化处理,使其像素值的均值为0,方差为1。对于光流图像,我们只进行了像素值的归一化处理,使其像素值的范围在[-1, 1]之间。最后,我们将图像转换为PyTorch张量,并将其作为输入传递给TwoStreamEarlyFusion模型。
阅读全文
相关推荐
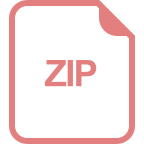
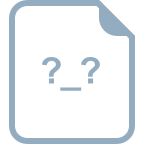


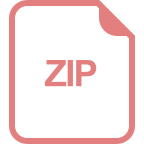
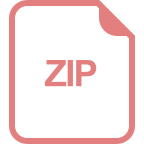
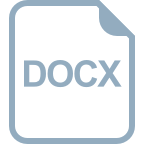
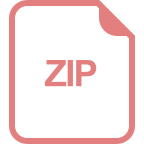
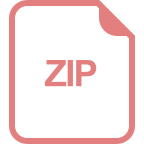
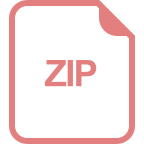
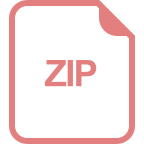
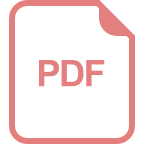
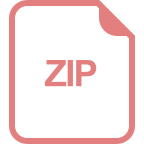
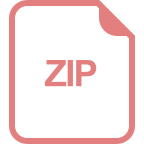
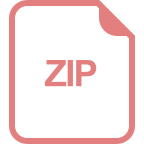