android实现了蓝牙连接怎样实现收发信息
时间: 2023-12-26 17:04:39 浏览: 48
你可以使用 Android 的 Bluetooth API 来实现蓝牙连接和数据收发。首先,你需要获取 BluetoothAdapter 对象并检查设备是否支持蓝牙功能。然后,你可以搜索和配对其他蓝牙设备,并使用 BluetoothSocket 在设备之间创建一个数据通道。最后,你可以使用 InputStream 和 OutputStream 对象来读取和发送数据。更多关于 Android 蓝牙开发的信息,你可以参考官方文档或者相应的教程。
相关问题
蓝牙收发文件android studio
要在Android Studio中实现蓝牙收发文件的功能,可以使用以下步骤:
1. 首先,确保手机是否支持蓝牙功能。可以使用以下代码检查蓝牙是否已打开:
```java
if (mBluetoothAdapter == null) {
Toast.makeText(getApplicationContext(), "无法打开手机蓝牙,请确认手机是否有蓝牙功能!", Toast.LENGTH_SHORT).show();
finish();
return;
}
```
2. 接下来,可以使用一个蓝牙库来简化蓝牙操作。例如,你可以使用一个名为"Android Studio 的蓝牙串口通信"的依赖库,它提供了方便的方法来实现蓝牙通信功能。
3. 在你的布局文件中添加一个按钮用于连接蓝牙设备:
```xml
<Button
android:id="@+id/connectButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="连接" />
```
4. 在你的Java代码中,为按钮添加点击事件处理程序,并在其中实现连接和断开蓝牙设备的逻辑:
```java
final Button connectButton = findViewById(R.id.connectButton);
connectButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (mBluetoothAdapter.isEnabled() == false) {
Toast.makeText(getApplicationContext(), "请先打开蓝牙", Toast.LENGTH_LONG).show();
return;
}
if (mBluetoothSocket == null) {
// 如果未连接设备,则打开DevicesListActivity搜索设备
Intent serveIntent = new Intent(getApplicationContext(), DevicesListActivity.class);
startActivityForResult(serveIntent, 1); // 设置返回宏定义
} else {
// 关闭连接socket
try {
bRun = false;
Thread.sleep(2000);
is.close();
mBluetoothSocket.close();
mBluetoothSocket = null;
connectButton.setText("连接");
} catch (InterruptedException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
});
```
以上是在Android Studio中实现蓝牙收发文件的基本步骤。你可以根据需要进一步完善和扩展这些功能。
Android 蓝牙连接多个设备
在 Android 中,可以通过 BluetoothAdapter 类和 BluetoothDevice 类来实现蓝牙设备的连接。要连接多个蓝牙设备,可以通过以下步骤实现:
1. 扫描周围的蓝牙设备,获取需要连接的蓝牙设备的 BluetoothDevice 对象;
2. 通过 BluetoothAdapter 的 connectGatt() 方法连接指定的蓝牙设备;
3. 在 BluetoothGattCallback 的回调方法中处理连接状态的变化和收发数据等操作。
注意,每个 BluetoothDevice 对象只能连接一个蓝牙设备,如果需要连接多个设备,需要创建多个 BluetoothDevice 对象并分别连接。
以下是一个简单的示例代码,演示如何连接两个蓝牙设备:
```java
// 获取 BluetoothAdapter 对象
BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
// 获取需要连接的两个蓝牙设备的 BluetoothDevice 对象
BluetoothDevice device1 = bluetoothAdapter.getRemoteDevice("device1_address");
BluetoothDevice device2 = bluetoothAdapter.getRemoteDevice("device2_address");
// 连接第一个设备
BluetoothGatt gatt1 = device1.connectGatt(context, false, gattCallback1);
// 连接第二个设备
BluetoothGatt gatt2 = device2.connectGatt(context, false, gattCallback2);
```
其中,gattCallback1 和 gattCallback2 分别为连接两个设备的 BluetoothGattCallback 对象,用于处理连接状态的变化和收发数据等操作。需要注意的是,如果需要同时连接多个设备,需要在 BluetoothGattCallback 的回调方法中判断连接状态和设备的 UUID 等信息,以区分不同的设备。
相关推荐
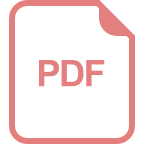
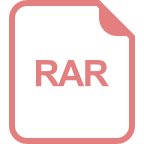
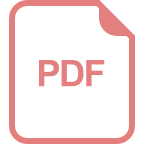
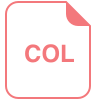
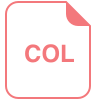










