设计4个类:Person,Student,Employee和TeachingAssistant,并根据main()函数能输出样例内容 Person类中包括数据成员string name,函数void introduce()输入内容cout << "My name is " << name << ". " << endl;) Student类继承自Person类,包括新数据成员int studentID, 继承的void introduce()输入内容包括cout << "My name is " << name << ". " << endl;cout << "I am a student. My student ID is " << studentID << "." << endl; Employee类继承自Person类,包括新数据成员string company 继承的void introduce()输入内容包括cout << "My name is " << name << ". " << endl;cout << "I am an employee. I work at " << company << "." << endl; TeachingAssistant类继承自Student类和Employee 继承的void introduce()输入内容包括cout << "My name is " << name << ". " << endl;cout << "I am a teaching assistant. I work at " << company << " and my student ID is " << studentID << "." <<endl;
时间: 2023-07-16 15:11:51 浏览: 98
以下是代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Person {
protected:
string name;
public:
Person(string n) : name(n) {}
void introduce() {
cout << "My name is " << name << ". " << endl;
}
};
class Student : public Person {
private:
int studentID;
public:
Student(string n, int id) : Person(n), studentID(id) {}
void introduce() {
cout << "My name is " << name << ". " << endl;
cout << "I am a student. My student ID is " << studentID << "." << endl;
}
};
class Employee : public Person {
private:
string company;
public:
Employee(string n, string c) : Person(n), company(c) {}
void introduce() {
cout << "My name is " << name << ". " << endl;
cout << "I am an employee. I work at " << company << "." << endl;
}
};
class TeachingAssistant : public Student, public Employee {
public:
TeachingAssistant(string n, int id, string c) : Student(n, id), Employee(n, c) {}
void introduce() {
cout << "My name is " << name << ". " << endl;
cout << "I am a teaching assistant. I work at " << company << " and my student ID is " << studentID << "." << endl;
}
};
int main() {
Person p("Tom");
p.introduce();
Student s("Alice", 123);
s.introduce();
Employee e("Bob", "ABC Company");
e.introduce();
TeachingAssistant t("Chris", 456, "XYZ University");
t.introduce();
return 0;
}
```
输出结果为:
```
My name is Tom.
My name is Alice.
I am a student. My student ID is 123.
My name is Bob.
I am an employee. I work at ABC Company.
My name is Chris.
I am a teaching assistant. I work at XYZ University and my student ID is 456.
```
阅读全文
相关推荐
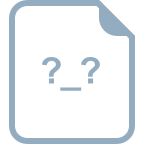
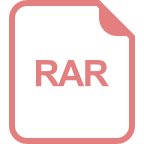
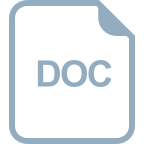

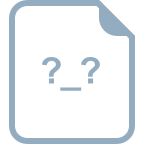
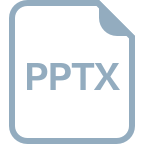
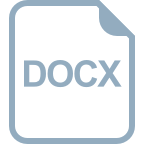
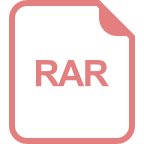




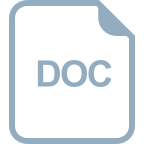
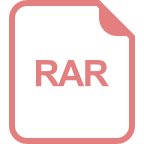
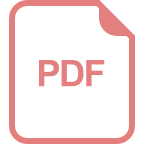
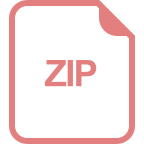
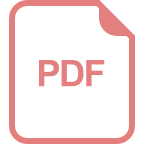
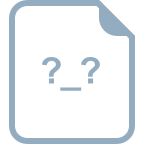
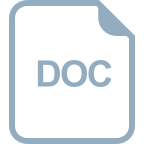