编 写 方 法 public static void bubbleSort( int[]a)对数组a采用冒泡法排序 (从小到大) 。在main方法中定义一整型数组(用数据{10,8,21,65,32,51,74,14,28,95}进行初始化) 对排序方法进行测试, 并输出结果。
时间: 2024-12-12 22:25:42 浏览: 5
在Java中,你可以按照以下步骤编写一个`bubbleSort`方法,使用冒泡排序算法对整数数组进行升序排列:
```java
public class BubbleSortExample {
// 定义公共静态方法,接受一个int类型的数组作为参数
public static void bubbleSort(int[] a) {
int n = a.length;
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
// 如果当前元素大于下一个元素,则交换它们
if (a[j] > a[j + 1]) {
// 交换操作
int temp = a[j];
a[j] = a[j + 1];
a[j + 1] = temp;
}
}
}
}
// 主方法
public static void main(String[] args) {
// 初始化整型数组
int[] array = {10, 8, 21, 65, 32, 51, 74, 14, 28, 95};
System.out.println("Original Array:");
for (int num : array) {
System.out.print(num + " ");
}
System.out.println();
// 调用排序方法并打印排序后的数组
bubbleSort(array);
System.out.println("\nSorted Array in Ascending Order:");
for (int num : array) {
System.out.print(num + " ");
}
System.out.println();
}
}
```
当你运行这个程序,它会首先显示原始数组,然后经过冒泡排序后,输出排序后的数组。注意,冒泡排序不是最高效的排序算法,但对于小规模的数据,其性能是可以接受的。
阅读全文
相关推荐
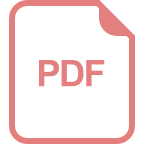
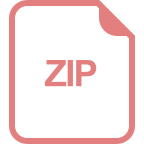















