esp32 flash读写
时间: 2023-09-24 19:07:25 浏览: 392
对于ESP32,您可以使用ESP-IDF(ESP32 IoT Development Framework)中提供的API来进行Flash的读写操作。
要进行Flash的读取,您可以使用 `spi_flash_read()` 函数。以下是一个示例代码,展示了如何读取Flash中的数据:
```c
#include <stdio.h>
#include <string.h>
#include "esp_system.h"
#include "esp_spi_flash.h"
void app_main()
{
// 读取Flash
uint32_t address = 0x10000; // Flash中的起始地址
uint32_t length = 4096; // 要读取的数据长度(以字节为单位)
uint8_t data[length]; // 存储读取的数据的缓冲区
esp_err_t err = spi_flash_read(address, (void*)&data, length);
if (err == ESP_OK)
{
printf("Flash read success!\n");
// 在这里可以对读取到的数据进行处理
}
else
{
printf("Flash read failed: %s\n", esp_err_to_name(err));
}
}
```
要进行Flash的写入,您可以使用 `spi_flash_write()` 函数。以下是一个示例代码,展示了如何将数据写入到Flash中:
```c
#include <stdio.h>
#include <string.h>
#include "esp_system.h"
#include "esp_spi_flash.h"
void app_main()
{
// 写入Flash
uint32_t address = 0x10000; // Flash中的起始地址
const uint8_t data[] = "Hello, ESP"; // 要写入的数据
esp_err_t err = spi_flash_write(address, (void*)&data, sizeof(data));
if (err == ESP_OK)
{
printf("Flash write success!\n");
}
else
{
printf("Flash write failed: %s\n", esp_err_to_name(err));
}
}
```
请注意,进行Flash操作需要在 `sysflash` 分区之外分配内存。还要确保地址和长度的设置正确,以避免出现读/写错误。
阅读全文
相关推荐
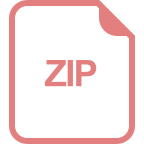
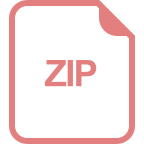















