C#中读取文件夹数量
时间: 2023-10-19 14:32:00 浏览: 297
要在C#中读取文件夹数量,可以使用`Directory.GetDirectories`方法来获取指定路径下的所有文件夹,并使用`Length`属性获取文件夹数量。以下是一个示例代码:
```csharp
using System;
using System.IO;
class Program
{
static void Main()
{
string path = @"C:\YourFolderPath";
string[] directories = Directory.GetDirectories(path);
int folderCount = directories.Length;
Console.WriteLine("Folder count: " + folderCount);
}
}
```
请注意将`C:\YourFolderPath`替换为你要读取文件夹数量的实际路径。运行上述代码将会打印出文件夹的数量。
相关问题
unity怎么读取文件夹中的文件数量
您好!关于您的问题,可以通过以下代码实现:
```c#
using System.IO;
using UnityEngine;
public class FileCount : MonoBehaviour
{
public string folderPath; //文件夹路径
// Start is called before the first frame update
void Start()
{
int fileCount = Directory.GetFiles(folderPath).Length;
Debug.Log("文件夹中的文件数量为:" + fileCount);
}
}
```
其中,Directory.GetFiles(folderPath) 方法可以获取文件夹中的所有文件路径,并通过 Length 属性获取数量。需要注意的是,folderPath 变量需要填写正确的文件夹路径。
希望能够帮助您解决问题。
c# 检索指定文件夹下所有字符串,获取"sfun.UpOperation"和“global”之间的字符串
你可以使用 C# 中的 `Directory` 和 `File` 类来遍历指定文件夹下的所有文件,然后使用 `StreamReader` 来读取文件内容。对于每个文件的内容,你可以使用正则表达式来查找指定的字符串。以下是一个示例代码:
```csharp
using System.IO;
using System.Text.RegularExpressions;
string folderPath = "your/folder/path";
string pattern = @"sfun\.UpOperation\(""([^""]*)""(?:(?!global).)*";
foreach (string filePath in Directory.GetFiles(folderPath))
{
using (StreamReader reader = new StreamReader(filePath))
{
string content = reader.ReadToEnd();
MatchCollection matches = Regex.Matches(content, pattern);
foreach (Match match in matches)
{
string value = match.Groups[1].Value;
Console.WriteLine(value);
}
}
}
```
这段代码会遍历指定文件夹下的所有文件,读取每个文件的内容,并使用正则表达式 `@"sfun\.UpOperation\(""([^""]*)""(?:(?!global).)*"` 查找所有匹配的字符串。其中,`([^"]*)` 表示匹配零个或多个非双引号字符,这样就可以获取在 `sfun.UpOperation(` 和 `)` 之间的字符串。`(?:(?!global).)*` 表示匹配任意数量的字符,但不包含 `global` 字符串。这样就可以确保匹配的字符串不包含 `global`。
阅读全文
相关推荐
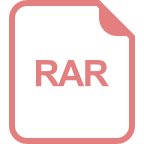
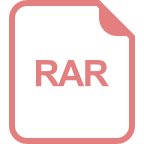
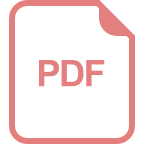
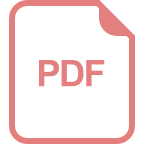
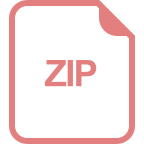
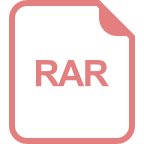
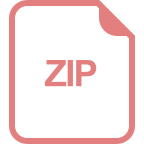
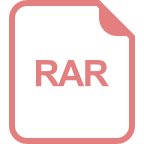
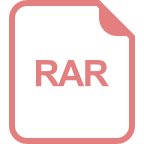
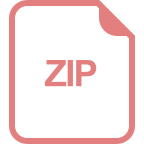
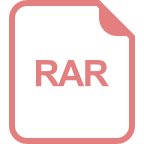
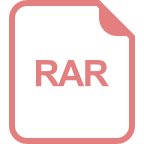
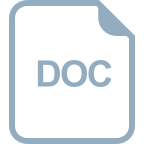
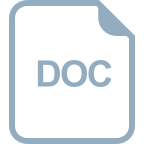