带旋转和缩放的形状匹配代码
时间: 2024-11-15 16:30:07 浏览: 12
带旋转和缩放的形状匹配通常涉及图像处理和计算机视觉技术,特别是在物体识别和计算机图形学领域。为了找到两个形状之间的对应关系,即使它们经历了旋转和缩放,你需要使用一些算法,比如特征检测、模板匹配、仿射变换或刚体变换(包括旋转变换和平移变换)。
以下是基本的步骤概述:
1. **特征提取**:从原始形状(模板)和目标图像中提取关键点或特征描述符,如SIFT、SURF、ORB或HOG等。
2. **预处理**:对目标图像应用旋转和缩放操作使其与模板对齐。这可能涉及到检测并应用逆变换到关键点上。
3. **匹配**:使用特征匹配算法(如Brute Force Matching、FLANN或KNN)比较模板和目标图像的关键点,寻找最相似的部分。
4. **校准**:结合特征匹配结果,确定最佳的旋转和缩放矩阵,可能是通过最小化特征点间的欧氏距离或其他相似度度量。
5. **验证**:检查匹配的质量,可能需要设置阈值或使用非极大值抑制(Non-Maximum Suppression)来排除错误匹配。
以下是一个简化版的Python示例(使用OpenCV库),展示如何进行基础的特征匹配:
```python
import cv2
import numpy as np
def match_shapes(template, image, scale=1.0, rotation=0):
# 使用SIFT提取特征
sift = cv2.xfeatures2d.SIFT_create()
keypoints_t, descriptors_t = sift.detectAndCompute(template, None)
keypoints_i, descriptors_i = sift.detectAndCompute(image, None)
# 使用BFMatcher进行匹配
matcher = cv2.BFMatcher()
matches = matcher.knnMatch(descriptors_t, descriptors_i, k=2)
# 应用几何校正
good_matches = []
for m, n in matches:
if m.distance < 0.75 * n.distance:
good_matches.append(m)
if len(good_matches) > 4:
src_pts = np.float32([keypoints_t[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
dst_pts = np.float32([keypoints_i[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
h, w = template.shape[:2]
warped_image = cv2.warpPerspective(image, M, (int(w*scale), int(h*scale)), flags=cv2.INTER_LINEAR)
# 对比原图和变形后的图像,看是否匹配良好
# ...
return warped_image, M
# 示例用法
template, image = ... # 提供模板图片和待匹配图片
warped_image, transform = match_shapes(template, image, scale=1.2, rotation=10)
```
阅读全文
相关推荐








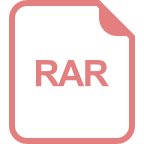








