#include <stdio.h> #include <iostream> #include <chrono> #include <thread> #include <DjiRtspImageSource.h> #define STB_IMAGE_WRITE_IMPLEMENTATION #include "stb_image_write.h" static inline int64_t now() { return std::chrono::duration_cast<std::chrono::milliseconds>(std::chrono::system_clock::now().time_since_epoch()).count(); } static int write_data_to_file(const char* name, uint8_t* data, int size) { FILE* fd = fopen(name, "wb"); if(fd) { int w = (int)fwrite(data, 1, size, fd); fclose(fd); return w; } else { return -1; } } char rtsp_url = "rtsp://192.168.42.142:8554/live"; int main(int argc, char** argv) { if(argc < 1) return -1; if(argc == 1) { std::cout << "Usage : " << argv[0] << " <url>" << std::endl; return -1; } int64_t ts = now(); DjiRtspImageSource service(rtsp_url); service.setImageCallback(nullptr, [&ts](void* handler, uint8_t* frmdata, int frmsize, int width, int height, int pixfmt) -> void { printf("Image %d@%p -- %dx%d -- %d\n", frmsize, frmdata, width, height, pixfmt); if(frmdata) { int64_t t = now(); if(t - ts > 1000) { ts = t; char name[64]; static int counter = 0; sprintf(name, "pictures/%dx%d-%d_%d.jpg", width, height, pixfmt, ++counter); if(pixfmt == 5) stbi_write_jpg(name, width, height, 3, frmdata, 80); } } }); service.start(); for(;;) //for(int i=0; i<30; i++) { std::this_thread::sleep_for(std::chrono::milliseconds(1000)); } service.stop(); std::cout << "done." << std::endl; return 0; } 上述c++代码帮我同等转换成python代码
时间: 2024-04-16 18:28:57 浏览: 12
你好!以下是将上述 C++ 代码转换为 Python 代码的等效版本:
```python
import cv2
import time
rtsp_url = "rtsp://192.168.42.142:8554/live"
def write_data_to_file(name, data):
with open(name, "wb") as fd:
fd.write(data)
return len(data)
return -1
def image_callback(handler, frmdata, frmsize, width, height, pixfmt):
print(f"Image {frmsize}@{frmdata} -- {width}x{height} -- {pixfmt}")
if frmdata:
t = int(time.time() * 1000)
if t - ts > 1000:
ts = t
name = f"pictures/{width}x{height}-{pixfmt}_{counter}.jpg"
if pixfmt == 5:
write_data_to_file(name, frmdata)
def main(rtsp_url):
ts = int(time.time() * 1000)
service = cv2.VideoCapture(rtsp_url)
counter = 0
while True:
ret, frame = service.read()
if not ret:
break
t = int(time.time() * 1000)
if t - ts > 1000:
ts = t
counter += 1
name = f"pictures/{frame.shape[1]}x{frame.shape[0]}-5_{counter}.jpg"
cv2.imwrite(name, frame, [int(cv2.IMWRITE_JPEG_QUALITY), 80])
service.release()
print("done.")
if __name__ == "__main__":
main(rtsp_url)
```
请注意,上述 Python 代码使用了 `cv2` 库来处理视频流和图像。确保在运行代码之前,已经安装了相应的库。此外,Python 代码中的图像保存路径 `pictures/` 需要事先存在。
相关推荐
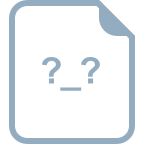
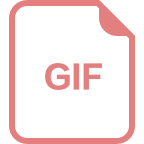
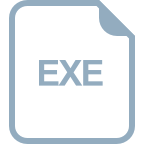















