nodejs axios 调用某个函数,函数里有异步操作,如何等待函数返回结果再进行下一步操作
时间: 2024-04-21 10:22:36 浏览: 39
在 Node.js 中,可以使用 async/await 或者 Promise 来等待函数返回结果再进行下一步操作。
使用 async/await 的示例代码如:
```javascript
const axios = require('axios');
async function fetchData() {
try {
const response = await axios.get('https://api.example.com/data');
// 在这里处理返回的数据
console.log(response.data);
// 进行下一步操作
console.log('继续执行其他操作');
} catch (error) {
// 处理错误
console.error(error);
}
}
fetchData();
```
在上述代码中,`fetchData` 函数是一个异步函数,使用 `await` 关键字等待 `axios.get` 方法返回结果。如果请求成功,可以在 `response` 对象中获取返回的数据,然后进行下一步操作。如果请求失败,可以在 `catch` 块中处理错误。
另一种方式是使用 Promise 链式调用的方式:
```javascript
const axios = require('axios');
function fetchData() {
return axios.get('https://api.example.com/data')
.then(response => {
// 在这里处理返回的数据
console.log(response.data);
// 进行下一步操作
console.log('继续执行其他操作');
})
.catch(error => {
// 处理错误
console.error(error);
});
}
fetchData();
```
在这种方式中,`fetchData` 函数返回一个 Promise 对象,可以通过 `then` 方法处理成功的回调函数,通过 `catch` 方法处理失败的回调函数。
无论是使用 async/await 还是 Promise,都可以等待异步操作完成后再进行下一步操作。
相关推荐
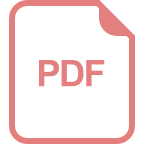
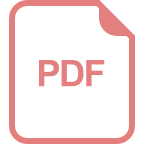















