分别写出在散列表中插入和删除关键字为k的一个记录的算法C语言,解决冲突的方法为链地址法
时间: 2024-10-10 12:14:09 浏览: 69
在使用链地址法解决散列表冲突的情况下,C语言中插入和删除关键字为k的记录的简单算法可以如下所示:
**插入操作(Insertion):**
```c
typedef struct Node {
int key; // 关键字
int value; // 记录值
struct Node* next; // 指向下一个节点的指针
} HashNode;
// 假设hash_size是哈希表的大小
void insert(int k, int value, HashNode** hash_table, int hash_func) {
int index = hash_func(k, hash_size); // 使用哈希函数计算索引
HashNode* new_node = (HashNode*)malloc(sizeof(HashNode));
if (!new_node) { // 如果内存分配失败
printf("Memory allocation failed.\n");
return;
}
new_node->key = k;
new_node->value = value;
new_node->next = *hash_table[index]; // 初始时指向空
(*hash_table)[index] = new_node; // 将新节点插入到对应位置
}
```
**删除操作(Deletion):**
```c
void delete(int k, HashNode** hash_table, int hash_func) {
int index = hash_func(k, hash_size);
HashNode* current = *hash_table[index];
if (current == NULL) {
printf("Key not found in the table.\n");
return;
}
if (current->key == k) {
HashNode* temp = current;
*hash_table[index] = current->next; // 删除当前节点,并将下一个节点移动到位
free(temp); // 释放已删除的节点内存
} else {
HashNode* prev = current;
while (prev->next != NULL && prev->next->key != k) {
prev = prev->next;
}
if (prev->next != NULL) {
prev->next = prev->next->next; // 跳过找到的节点并链接剩余部分
} else {
printf("Key not found in the chain.\n");
}
}
}
```
阅读全文
相关推荐

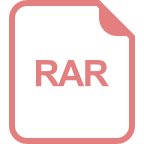
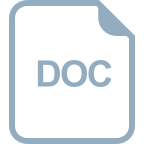
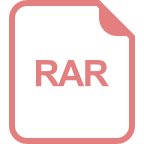
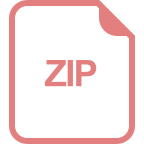
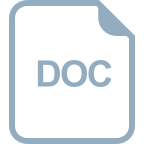
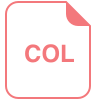
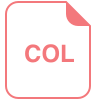
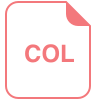
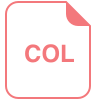
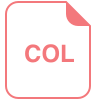

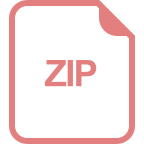