使用单片机AT89C51进行温度报警器代码
时间: 2024-03-09 10:48:59 浏览: 15
以下是一个基于AT89C51单片机的温度报警器代码,当温度超过设定的阈值时,会触发报警器。
```c
#include <reg51.h>
#define DATA_PORT P0
sbit SDA = P2^0; // I2C数据线
sbit SCL = P2^1; // I2C时钟线
void delay(int t) // 延时函数
{
int i, j;
for (i = 0; i < t; i++) {
for (j = 0; j < 100; j++);
}
}
void i2c_start() // I2C起始信号
{
SDA = 1;
SCL = 1;
delay(1);
SDA = 0;
delay(1);
SCL = 0;
}
void i2c_stop() // I2C停止信号
{
SDA = 0;
delay(1);
SCL = 1;
delay(1);
SDA = 1;
}
unsigned char i2c_write(unsigned char dat) // I2C写数据
{
unsigned char i, ack;
for (i = 0; i < 8; i++) {
if (dat & 0x80) {
SDA = 1;
} else {
SDA = 0;
}
delay(1);
SCL = 1;
delay(1);
SCL = 0;
dat <<= 1;
}
SDA = 1;
delay(1);
SCL = 1;
delay(1);
ack = SDA;
SCL = 0;
return ack;
}
unsigned char i2c_read() // I2C读数据
{
unsigned char i, dat;
for (i = 0; i < 8; i++) {
SDA = 1;
delay(1);
SCL = 1;
delay(1);
dat <<= 1;
if (SDA) {
dat |= 0x01;
}
SCL = 0;
}
return dat;
}
unsigned char ds18b20_init() // DS18B20初始化
{
unsigned char i;
DATA_PORT = 0xff;
delay(100);
DATA_PORT = 0x00;
delay(500);
DATA_PORT = 0xff;
delay(100);
i = DATA_PORT;
delay(500);
return i;
}
void ds18b20_write(unsigned char dat) // DS18B20写数据
{
unsigned char i;
for (i = 0; i < 8; i++) {
DATA_PORT = 0x00;
delay(2);
if (dat & 0x01) {
DATA_PORT = 0xff;
} else {
DATA_PORT = 0x00;
}
delay(60);
DATA_PORT = 0xff;
dat >>= 1;
}
}
unsigned char ds18b20_read() // DS18B20读数据
{
unsigned char i, dat;
for (i = 0; i < 8; i++) {
DATA_PORT = 0x00;
delay(2);
DATA_PORT = 0xff;
delay(10);
dat >>= 1;
if (DATA_PORT) {
dat |= 0x80;
}
delay(50);
}
return dat;
}
void ds18b20_convert() // DS18B20温度转换
{
ds18b20_init();
ds18b20_write(0xcc);
ds18b20_write(0x44);
}
unsigned int ds18b20_read_temperature() // DS18B20读温度
{
unsigned char temp1, temp2;
unsigned int temp;
ds18b20_init();
ds18b20_write(0xcc);
ds18b20_write(0xbe);
temp1 = ds18b20_read();
temp2 = ds18b20_read();
temp = temp2;
temp <<= 8;
temp |= temp1;
return temp;
}
void i2c_write_byte(unsigned char addr, unsigned char dat) // I2C写一个字节
{
i2c_start();
i2c_write(0x90);
i2c_write(addr);
i2c_write(dat);
i2c_stop();
}
unsigned char i2c_read_byte(unsigned char addr) // I2C读一个字节
{
unsigned char dat;
i2c_start();
i2c_write(0x90);
i2c_write(addr);
i2c_start();
i2c_write(0x91);
dat = i2c_read();
i2c_stop();
return dat;
}
void main()
{
unsigned int temp;
unsigned char temp1, temp2;
unsigned char alarm = 0;
i2c_start();
i2c_write_byte(0x01, 0x00); // 温度阈值:25°C
i2c_stop();
while (1) {
ds18b20_convert();
delay(500);
temp = ds18b20_read_temperature();
temp1 = temp >> 8;
temp2 = temp & 0xff;
i2c_write_byte(0x00, temp1); // 写入温度高位
i2c_write_byte(0x01, temp2); // 写入温度低位
if (temp > 250) { // 温度超过阈值
if (!alarm) { // 如果还没有报警
P1 = 0x01; // 报警器响
alarm = 1; // 标记已经报警
}
} else {
P1 = 0x00; // 关闭报警器
alarm = 0; // 标记没有报警
}
delay(500);
}
}
```
在这个代码中,我们使用了DS18B20数字温度传感器来测量环境温度,并且使用了I2C总线来与EEPROM进行通信,将温度值和温度阈值写入EEPROM中,实现了温度报警功能。当温度超过设定的阈值时,会触发报警器。
相关推荐
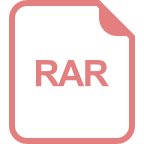
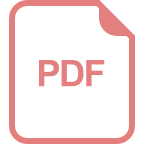
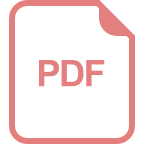














