如何在Vue3项目中使用POST方法实现与帆软报表系统的跨域数据传输,并将帆软报表页面嵌入到Web页面并解决跨域问题?
时间: 2024-11-29 08:33:00 浏览: 0
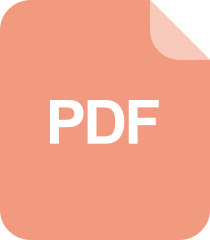
如何在vue项目中嵌入jsp页面的方法(2种)
在Vue3项目中,要通过POST方法与帆软报表系统进行跨域数据传输,并解决跨域问题,可以按照以下步骤操作:
1. **配置axios或fetch请求**:
- 如果你在项目中使用了`axios`库,可以在创建axios实例时设置`proxyTable`属性来处理跨域。例如,在`main.js`中:
```javascript
import axios from 'axios';
const instance = axios.create({
baseURL: process.env.BASE_API,
withCredentials: true, // 如果需要发送cookie
headers: {
'Content-Type': 'application/json', // 根据实际需求设置
},
proxy: { // 允许跨域
'/report/*': {
target: 'http://your-fanyun-report-api.com',
changeOrigin: true,
pathRewrite: {
'^/report': '', // 将请求路径替换为实际API路径
},
},
},
});
```
- 或者使用浏览器的`fetch` API,你需要在服务器端设置响应头允许跨域,如Node.js的Express应用:
```javascript
app.use((req, res, next) => {
if (req.method === 'OPTIONS') {
res.header('Access-Control-Allow-Origin', '*');
res.header('Access-Control-Allow-Methods', 'GET, POST, OPTIONS');
res.header('Access-Control-Allow-Headers', 'Content-Type, Authorization');
res.status(204).end();
} else {
next();
}
});
```
2. **在组件内部发起请求**:
使用配置好的axios或fetch实例发起POST请求:
```javascript
async sendData() {
try {
let response = await axios.post('/report/data', yourData);
console.log(response.data);
} catch (error) {
console.error(error);
}
}
```
3. **帆软报表嵌入**:
- 获取报表的HTML代码通常有专门的API提供,你可以从帆软报表系统的后台获取报表ID,然后拼接URL(假设是`http://fanyun-report.com/getReport?reportId=your-report-id`)。
- 将这个HTML片段插入到 Vue 页面中的某个容器元素中:
```html
<div v-html="reportHtml"></div>
```
- 然后在Vue组件中动态绑定这个值:
```javascript
data() {
return {
reportHtml: '',
};
},
mounted() {
this.getReport();
},
methods: {
getReport() {
axios.get('/report/get', {
params: {
reportId: 'your-report-id',
},
})
.then(response => {
this.reportHtml = response.data;
})
.catch(error => {
console.error(error);
});
},
},
```
阅读全文
相关推荐
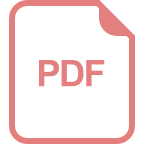
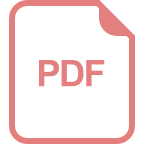
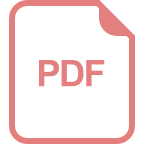
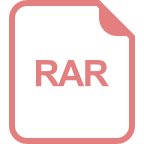
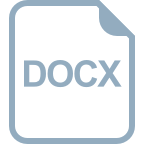
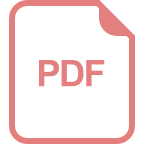
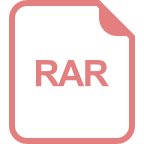
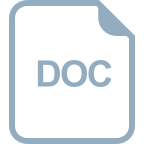
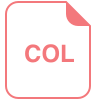








