通过C#类库绘制正态分布的统计图(通用)
时间: 2023-07-12 08:05:21 浏览: 471
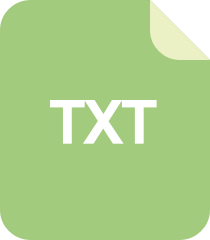
C#绘制曲线图通用方法

可以使用C#的类库Chart来绘制正态分布的统计图。下面是一个简单的示例代码,用于绘制正态分布曲线:
```csharp
using System;
using System.Windows.Forms.DataVisualization.Charting;
namespace NormalDistributionChart
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
DrawChart();
}
private void DrawChart()
{
// Create a new chart object
Chart chart = new Chart();
// Set the chart area
ChartArea area = new ChartArea();
chart.ChartAreas.Add(area);
// Add the series
Series series = new Series();
series.ChartType = SeriesChartType.Line;
series.Color = System.Drawing.Color.Blue;
chart.Series.Add(series);
// Set the chart title
chart.Titles.Add("Normal Distribution");
// Set the x-axis and y-axis labels
chart.ChartAreas[0].AxisX.Title = "X";
chart.ChartAreas[0].AxisY.Title = "Y";
// Set the x-axis range
double xMin = -4;
double xMax = 4;
chart.ChartAreas[0].AxisX.Minimum = xMin;
chart.ChartAreas[0].AxisX.Maximum = xMax;
// Set the y-axis range
double yMin = 0;
double yMax = 0.5;
chart.ChartAreas[0].AxisY.Minimum = yMin;
chart.ChartAreas[0].AxisY.Maximum = yMax;
// Calculate the normal distribution values
double[] xValues = new double[100];
double[] yValues = new double[100];
double mean = 0;
double stdDev = 1;
double step = (xMax - xMin) / (xValues.Length - 1);
for (int i = 0; i < xValues.Length; i++)
{
xValues[i] = xMin + step * i;
yValues[i] = Math.Exp(-Math.Pow(xValues[i] - mean, 2) / (2 * Math.Pow(stdDev, 2))) / (stdDev * Math.Sqrt(2 * Math.PI));
}
// Add the data to the chart
chart.Series[0].Points.DataBindXY(xValues, yValues);
// Add the chart to the form
this.Controls.Add(chart);
chart.Dock = DockStyle.Fill;
}
}
}
```
在这个示例中,我们使用Chart类库创建了一个新的图表对象,并且设置了图表的标题、坐标轴标签、坐标轴范围等属性。然后,我们计算正态分布的概率密度函数,并将其作为数据添加到图表中。
最后,我们将图表添加到窗体控件中并进行显示。运行程序后,应该会看到一个正态分布曲线的统计图。
阅读全文
相关推荐
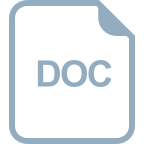
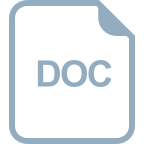
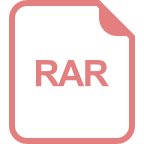
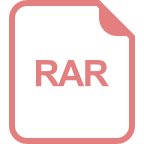
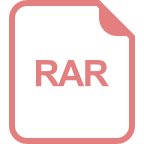
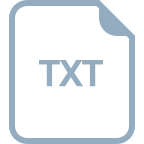
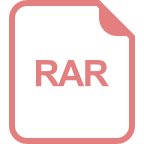
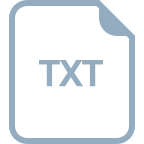
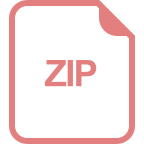
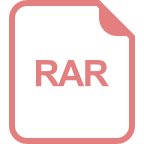
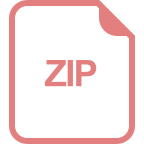
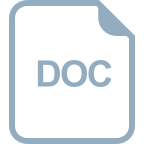
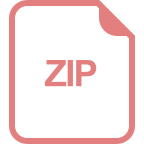
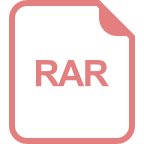
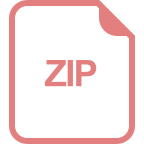
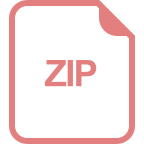