list<Employee> list2; for(list<Employee>::iterator it = list1.begin();it != list1.end();++it) { list<Employee>::iterator result = find_if(it,list1.end(),com_10000); if(result != list1.end()) list2.push_back(*result); } cout<<"salary is more than 10000:"<<endl; show(list2);
时间: 2024-02-11 13:07:51 浏览: 110
这段代码使用了 `find_if` 算法来查找 `list1` 中大于 10000 的元素,并将它们放到 `list2` 中。需要注意的是,这里的 `find_if` 函数的第二个参数应该是 `list1.end()` 而不是 `it++`。
另外,代码中使用了 `show` 函数来输出 `list2` 中的元素,但是该函数的实现没有给出。如果在代码中使用了该函数,需要先定义该函数来输出元素。
下面是经过修改后的代码:
```c++
list<Employee> list2;
for (auto it = list1.begin(); it != list1.end(); ++it) {
auto result = std::find_if(it, list1.end(), [](const Employee& e) {
return e.salary > 10000;
});
if (result != list1.end()) {
list2.push_back(*result);
}
}
cout << "salary is more than 10000:" << endl;
for (const auto& e : list2) {
// 输出 list2 中的元素
cout << e.name << " " << e.salary << endl;
}
```
这样可以将 `list1` 中大于 10000 的元素放到 `list2` 中,并输出 `list2` 中的元素。
相关问题
for(list<Employee>::iterator it = list1.begin();it != list1.end();++it) { list<Employee>::iterator result = find_if(it,it++,com_10000); list2.push_back(*result); }想要实现把list1中大于10000的数放到list2中
这段代码有一些问题,首先是 `it++` 在 `find_if` 函数中被使用,这样会导致 `it` 和 `result` 指向不同的元素,因此会把不符合条件的元素加入到 `list2` 中。其次,没有给出 `com_10000` 的定义,这可能导致无法正确判断元素是否大于 10000。最后,这段代码只能处理 `Employee` 类型的元素,不能处理其他类型。
如果要实现把 `list1` 中大于 10000 的元素放到 `list2` 中,可以使用以下代码:
```c++
for (auto it = list1.begin(); it != list1.end(); ++it) {
if (*it > 10000) {
list2.push_back(*it);
}
}
```
这样可以避免上述问题,并且可以处理任何类型的元素。
阅读全文
相关推荐
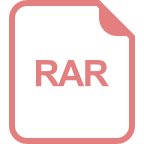
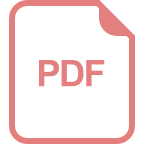
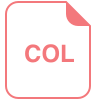
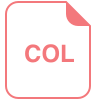
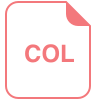
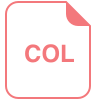
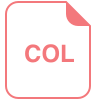
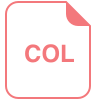
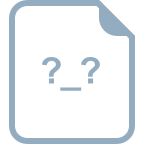
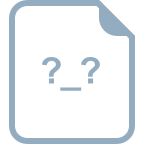