MFC 解析PDF生成单色位图
时间: 2024-01-16 22:03:38 浏览: 235
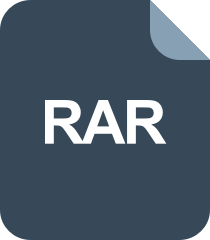
基于MFC的单文档画图

MFC(Microsoft Foundation Class)是微软公司提供的一套C++类库,用于开发Windows应用程序。PDF(Portable Document Format)是一种跨平台的文档格式,可以在不同设备和操作系统上显示和打印。本文将介绍如何使用MFC解析PDF文件,并生成单色位图。
1. 安装PDFium库
PDFium是一个开源的PDF解析库,可以用于解析PDF文件并提取文本、图像等内容。在使用MFC解析PDF文件之前,需要先安装PDFium库。可以从GitHub上下载PDFium源代码,并编译生成静态库或动态链接库。
2. 打开PDF文件
使用MFC打开PDF文件可以使用CFile类和CFileException类。首先使用CFile类打开PDF文件,代码如下:
CFile pdfFile;
if (!pdfFile.Open(_T("test.pdf"), CFile::modeRead | CFile::typeBinary))
{
AfxMessageBox(_T("Failed to open PDF file!"));
return;
}
如果PDF文件打开失败,会弹出一个错误提示框。如果文件打开成功,则可以从文件中读取PDF数据。
3. 解析PDF文件
使用PDFium库解析PDF文件可以使用FPDF_LoadDocument函数和FPDF_CloseDocument函数。首先使用FPDF_LoadDocument函数加载PDF文件,代码如下:
FPDF_DOCUMENT pdfDoc = FPDF_LoadDocument(pdfFile.GetFilePath(), nullptr);
如果PDF文件加载失败,可以使用FPDF_GetLastError函数获取错误信息。如果PDF文件加载成功,则可以使用FPDF_GetPageCount函数获取PDF页面数,并使用FPDF_LoadPage函数加载PDF页面。
4. 渲染PDF页面
使用PDFium库渲染PDF页面可以使用FPDF_RenderPageBitmap函数。首先创建一个位图对象,代码如下:
CBitmap bmp;
bmp.CreateBitmap(width, height, 1, 1, nullptr);
其中width和height是位图的宽度和高度,1表示每个像素占用1位(即单色位图),nullptr表示位图数据为空。然后使用FPDF_RenderPageBitmap函数将PDF页面渲染到位图中,代码如下:
BITMAP bmpInfo;
bmp.GetBitmap(&bmpInfo);
int stride = ((bmpInfo.bmWidth + 31) / 32) * 4;
BYTE* buffer = new BYTE[stride * bmpInfo.bmHeight];
FPDF_RenderPageBitmap(bitmap, pdfPage, 0, 0, bmpInfo.bmWidth, bmpInfo.bmHeight, 0, buffer, stride);
其中stride是位图每行数据的字节数,buffer是位图数据缓冲区。渲染完成后,可以将位图保存成BMP、PNG等格式的文件。
5. 关闭PDF文件
使用PDFium库关闭PDF文件可以使用FPDF_CloseDocument函数,代码如下:
FPDF_CloseDocument(pdfDoc);
6. 完整代码
下面是使用MFC解析PDF生成单色位图的完整代码:
#include "stdafx.h"
#include "pdfium/include/fpdfview.h"
#include "pdfium/include/fpdf_save.h"
void GenerateMonochromeBitmap(const CString& pdfFilePath, const CString& bmpFilePath, int width, int height)
{
CFile pdfFile;
if (!pdfFile.Open(pdfFilePath, CFile::modeRead | CFile::typeBinary))
{
AfxMessageBox(_T("Failed to open PDF file!"));
return;
}
FPDF_DOCUMENT pdfDoc = FPDF_LoadDocument(pdfFile.GetFilePath(), nullptr);
if (!pdfDoc)
{
AfxMessageBox(_T("Failed to load PDF document!"));
return;
}
int pageCount = FPDF_GetPageCount(pdfDoc);
if (pageCount == 0)
{
AfxMessageBox(_T("No pages found in PDF document!"));
FPDF_CloseDocument(pdfDoc);
return;
}
FPDF_PAGE pdfPage = FPDF_LoadPage(pdfDoc, 0);
if (!pdfPage)
{
AfxMessageBox(_T("Failed to load PDF page!"));
FPDF_CloseDocument(pdfDoc);
return;
}
CBitmap bmp;
bmp.CreateBitmap(width, height, 1, 1, nullptr);
BITMAP bmpInfo;
bmp.GetBitmap(&bmpInfo);
int stride = ((bmpInfo.bmWidth + 31) / 32) * 4;
BYTE* buffer = new BYTE[stride * bmpInfo.bmHeight];
FPDF_RenderPageBitmap(bitmap, pdfPage, 0, 0, bmpInfo.bmWidth, bmpInfo.bmHeight, 0, buffer, stride);
FPDF_ClosePage(pdfPage);
FPDF_CloseDocument(pdfDoc);
pdfFile.Close();
CImage image;
image.Create(bmpInfo.bmWidth, bmpInfo.bmHeight, 1, 0);
BYTE* dst = (BYTE*)image.GetBits();
BYTE* src = buffer;
for (int y = 0; y < bmpInfo.bmHeight; y++)
{
for (int x = 0; x < bmpInfo.bmWidth; x++)
{
int pixelIndex = x / 8;
int bitIndex = 7 - x % 8;
BYTE pixel = (src[pixelIndex] >> bitIndex) & 0x01;
dst[x] = pixel ? 0 : 255;
}
src += stride;
dst += bmpInfo.bmWidth;
}
image.Save(bmpFilePath, Gdiplus::ImageFormatPNG);
delete[] buffer;
}
int main()
{
CString pdfFilePath = _T("test.pdf");
CString bmpFilePath = _T("test.png");
GenerateMonochromeBitmap(pdfFilePath, bmpFilePath, 1280, 720);
return 0;
}
阅读全文
相关推荐
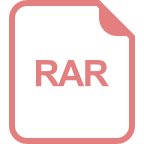
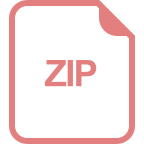
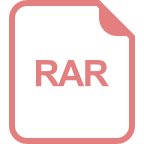
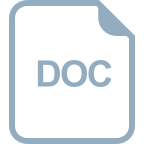
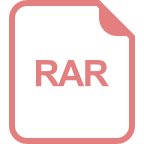
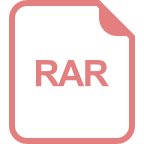
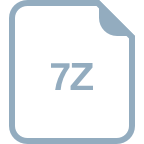
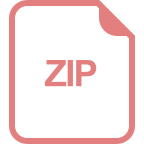
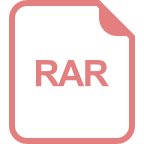
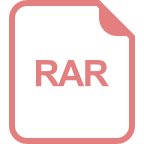
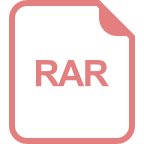