@ExceptionHandler(Throwable.class)
时间: 2023-04-09 22:04:41 浏览: 177
这是一个 Java 中的注解,用于处理在 Controller 层中未被捕获的异常。当 Controller 中出现未被处理的异常时,@ExceptionHandler(Throwable.class) 可以将异常信息返回给前端或者进行日志记录等操作。
相关问题
Java 环绕通知怎么拿到@ExceptionHandler(value = RuntimeException.class)的返回值
在 Java 的环绕通知中,可以使用 ProceedingJoinPoint 对象的 proceed() 方法来执行目标方法,并且可以获取目标方法的返回值。如果目标方法执行时抛出了异常,并且有对应的异常处理方法(如 @ExceptionHandler 注解所标注的方法),则可以在环绕通知中捕获该异常,并且调用异常处理方法返回的值。
以下是一个示例代码:
```java
@Around("execution(* com.example.MyController.*(..))")
public Object aroundAdvice(ProceedingJoinPoint joinPoint) throws Throwable {
Object result = null;
try {
result = joinPoint.proceed(); // 执行目标方法,获取返回值
} catch (Exception e) {
// 捕获目标方法抛出的异常,并且调用异常处理方法
MethodSignature signature = (MethodSignature) joinPoint.getSignature();
Method method = signature.getMethod();
if (method.isAnnotationPresent(ExceptionHandler.class)) {
ExceptionHandler exceptionHandler = method.getAnnotation(ExceptionHandler.class);
Class<?>[] exceptions = exceptionHandler.value();
for (Class<?> exception : exceptions) {
if (exception.isAssignableFrom(e.getClass())) {
Object handler = applicationContext.getBean(method.getDeclaringClass());
result = method.invoke(handler, e);
break;
}
}
} else {
throw e; // 没有对应的异常处理方法,继续抛出异常
}
}
return result;
}
```
在上述代码中,我们首先通过 ProceedingJoinPoint 对象的 proceed() 方法执行目标方法,并且获取目标方法的返回值。如果目标方法执行时抛出了异常,我们会捕获该异常,并且调用对应的异常处理方法。在调用异常处理方法时,我们需要使用反射来获取该方法,并且通过 invoke() 方法来调用该方法并且获取返回值。最终,我们将返回值返回给调用方。
springmvc SQL 异常 ExceptionHandler 无法捕捉
Spring MVC中的异常处理器`@ExceptionHandler`只能处理Controller层抛出的异常,而无法捕捉到DAO或Service层抛出的异常。
如果需要在DAO或Service层捕捉到SQL异常,并统一处理,可以采用以下两种方式:
1. 利用AOP拦截异常
可以使用Spring AOP拦截DAO或Service层的方法,在方法执行时捕捉异常,并进行统一处理。例如:
```java
@Aspect
@Component
public class ExceptionAspect {
@Autowired
private HttpServletResponse response;
@Around("execution(* com.example.dao.*.*(..)) || execution(* com.example.service.*.*(..))")
public Object handleException(ProceedingJoinPoint point) throws Throwable {
try {
return point.proceed();
} catch (SQLException e) {
response.sendError(HttpServletResponse.SC_INTERNAL_SERVER_ERROR, "SQL Exception");
} catch (Exception e) {
response.sendError(HttpServletResponse.SC_INTERNAL_SERVER_ERROR, "Unknown Exception");
}
return null;
}
}
```
2. 自定义异常并抛出
在DAO或Service层方法中捕捉到SQL异常后,可以将该异常包装成一个自定义异常,并抛出,然后在Controller层通过`@ExceptionHandler`捕捉到该自定义异常并进行统一处理。例如:
```java
// DAO或Service层中的方法
public void save(User user) throws SQLException, CustomException {
try {
// 执行SQL操作
} catch (SQLException e) {
throw new CustomException("SQL Exception", e);
}
}
// Controller层中的方法
@PostMapping("/user")
public void saveUser(@RequestBody User user) throws CustomException {
userService.save(user);
}
@ExceptionHandler(CustomException.class)
public void handleCustomException(HttpServletResponse response, CustomException e) throws IOException {
response.sendError(HttpServletResponse.SC_INTERNAL_SERVER_ERROR, e.getMessage());
}
```
通过以上两种方式,可以在DAO或Service层捕捉到SQL异常并进行统一处理。
阅读全文
相关推荐
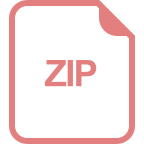
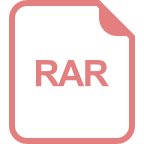
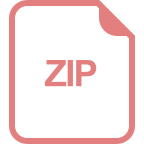
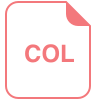
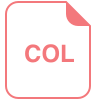
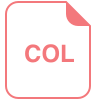
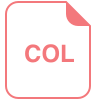




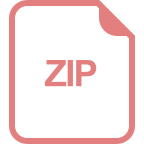
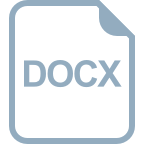