怎么用HashSet去重Java 对象
时间: 2023-07-18 17:15:45 浏览: 56
要使用HashSet去重Java对象,需要完成以下步骤:
1. 在Java类中重写hashCode()和equals()方法,以确保对象能够正确地比较和判定相等性。
2. 创建一个HashSet对象,该对象将用于存储不重复的Java对象。
3. 遍历Java对象的集合,将每个对象添加到HashSet中。
4. 最后,从HashSet中获取不重复的Java对象集合。
例如,假设有一个Student类,并且我们想要去除其中重复的对象。那么,我们需要在Student类中重写hashCode()和equals()方法,如下所示:
```
public class Student {
private String name;
private int age;
// constructor, getter and setter methods
@Override
public boolean equals(Object o) {
if (o == this) return true;
if (!(o instanceof Student)) {
return false;
}
Student student = (Student) o;
return age == student.age &&
Objects.equals(name, student.name);
}
@Override
public int hashCode() {
return Objects.hash(name, age);
}
}
```
然后,我们可以使用HashSet去重Java对象,如下所示:
```
Set<Student> students = new HashSet<>();
students.add(new Student("Alice", 20));
students.add(new Student("Bob", 22));
students.add(new Student("Alice", 20)); // This object is a duplicate and will not be added to the Set
```
最后,我们可以从HashSet中获取不重复的Java对象集合:
```
List<Student> uniqueStudents = new ArrayList<>(students);
```
相关推荐
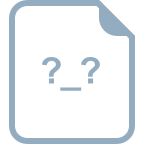














