用QT写一个链表
时间: 2023-07-04 21:07:32 浏览: 82
以下是一个基本的链表类实现,使用了QT中的QList类:
```cpp
#include <QtCore>
class Node {
public:
int data;
Node* next;
Node(int d) {
data = d;
next = nullptr;
}
};
class LinkedList {
public:
Node* head;
LinkedList() {
head = nullptr;
}
void append(int data) {
Node* new_node = new Node(data);
if (head == nullptr) {
head = new_node;
} else {
Node* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = new_node;
}
}
void print() {
Node* current = head;
while (current != nullptr) {
qDebug() << current->data;
current = current->next;
}
}
};
```
使用示例:
```cpp
LinkedList list;
list.append(1);
list.append(2);
list.append(3);
list.print();
```
输出:
```
1
2
3
```
阅读全文
相关推荐



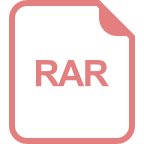
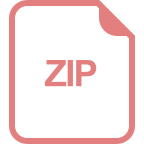
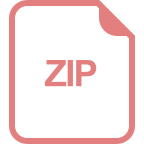
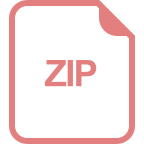
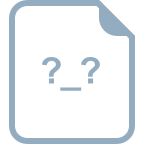