bottomnavigationview 中间凸起
时间: 2023-09-10 15:15:25 浏览: 205
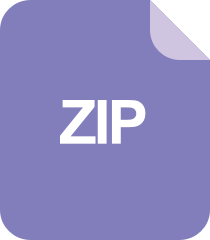
Android 实现底部导航中间菜单凸起效果
要实现 BottomNavigationView 中间凸起的效果,可以使用第三方库或者自定义控件实现。以下是自定义控件的实现方式:
1. 创建一个自定义控件类,继承自 BottomNavigationView。
2. 在自定义控件类的构造函数中,调用 init() 方法初始化控件。
3. 在 init() 方法中,设置 BottomNavigationView 的 ItemIconTintList、ItemTextColor 和 ItemBackgroundResource 属性,以及添加中间凸起的 Item。
4. 在自定义控件类中,重写 onMeasure() 方法,使中间凸起的 Item 占据 BottomNavigationView 的一定比例。
5. 在自定义控件类中,重写 onDraw() 方法,绘制中间凸起的 Item。
6. 在布局文件中使用自定义控件。
具体实现细节可以参考以下代码示例:
```java
public class CustomBottomNavigationView extends BottomNavigationView {
private static final int ITEM_SIZE = 5; // BottomNavigationView 的 Item 数量
private static final int MIDDLE_ITEM_INDEX = 2; // 中间凸起的 Item 索引
private static final int MIDDLE_ITEM_SIZE = 80; // 中间凸起的 Item 大小
private Paint mPaint;
private Path mPath;
public CustomBottomNavigationView(Context context) {
super(context);
init();
}
public CustomBottomNavigationView(Context context, AttributeSet attrs) {
super(context, attrs);
init();
}
public CustomBottomNavigationView(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
init();
}
private void init() {
// 设置 BottomNavigationView 的 ItemIconTintList、ItemTextColor 和 ItemBackgroundResource 属性
int[][] states = new int[][]{
new int[]{-android.R.attr.state_checked},
new int[]{android.R.attr.state_checked},
};
int[] colors = new int[]{
Color.GRAY,
Color.BLUE,
};
ColorStateList csl = new ColorStateList(states, colors);
setItemIconTintList(csl);
setItemTextColor(csl);
setBackgroundResource(R.color.white);
// 添加中间凸起的 Item
Menu menu = getMenu();
menu.add(Menu.NONE, R.id.item_middle, Menu.NONE, "")
.setIcon(R.drawable.ic_add)
.setShowAsAction(MenuItem.SHOW_AS_ACTION_ALWAYS);
// 初始化画笔和路径
mPaint = new Paint();
mPaint.setStyle(Paint.Style.FILL);
mPaint.setColor(getResources().getColor(R.color.white));
mPath = new Path();
}
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
super.onMeasure(widthMeasureSpec, heightMeasureSpec);
// 计算中间凸起的 Item 大小
int width = MeasureSpec.getSize(widthMeasureSpec);
int height = MeasureSpec.getSize(heightMeasureSpec);
int itemWidth = width / ITEM_SIZE;
int middleItemWidth = MIDDLE_ITEM_SIZE * (ITEM_SIZE - 2) / ITEM_SIZE;
int middleItemHeight = MIDDLE_ITEM_SIZE / 2;
// 设置中间凸起的 Item 大小
MenuItem middleItem = getMenu().findItem(R.id.item_middle);
middleItem.setIcon(R.drawable.ic_add);
middleItem.setWidth(middleItemWidth);
middleItem.setHeight(middleItemHeight);
// 设置中间凸起的 Item 占据 BottomNavigationView 的一定比例
ViewGroup.LayoutParams layoutParams = getLayoutParams();
layoutParams.height = height + middleItemHeight;
setLayoutParams(layoutParams);
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
// 绘制中间凸起的 Item
float centerX = getWidth() / ITEM_SIZE * MIDDLE_ITEM_INDEX;
float centerY = getHeight() / 2;
float radius = MIDDLE_ITEM_SIZE / 2;
mPath.reset();
mPath.moveTo(centerX - radius, centerY - radius);
mPath.lineTo(centerX + radius, centerY - radius);
mPath.lineTo(centerX, centerY + radius);
mPath.close();
canvas.drawPath(mPath, mPaint);
}
}
```
使用自定义控件时,只需在布局文件中引用即可:
```xml
<com.example.CustomBottomNavigationView
android:id="@+id/bottom_navigation_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:menu="@menu/bottom_navigation_menu" />
```
阅读全文
相关推荐
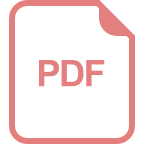
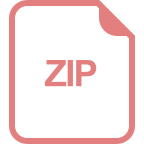
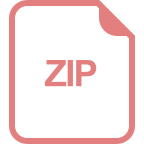
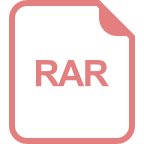
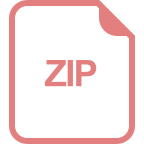
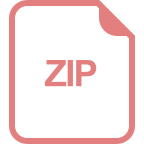
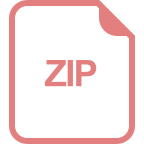
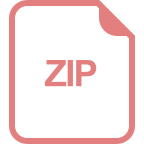


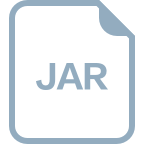
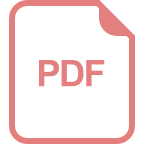
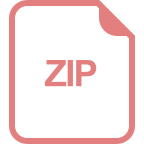
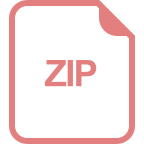
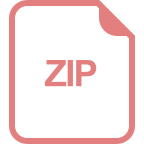
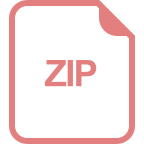