Inputs to a layer should be tensors. Got: <keras.engine.input_layer.InputLayer object at 0x00000237B0D43FD0>
时间: 2024-04-30 12:22:54 浏览: 11
This error occurs because you are trying to pass an InputLayer object to a layer that expects a tensor as input.
To fix this error, you need to make sure that you are passing tensors as inputs to the layers in your model. This can be done by calling the `output` attribute of the InputLayer object, which returns a tensor that can be passed to the next layer in the model.
For example, instead of passing the InputLayer object directly to the next layer, you would do:
```
input_layer = Input(shape=(input_shape,))
x = input_layer.output
x = Dense(units=64, activation='relu')(x)
...
```
Here, `input_layer.output` returns a tensor that is then passed to the Dense layer.
相关问题
Inputs to a layer should be tensors. Got: <keras.layers.
The error message suggests that the input to a Keras layer was not a tensor, which is required.
A tensor is a multi-dimensional array, similar to a matrix. In Keras, tensors are represented using the `tf.Tensor` class from the TensorFlow library.
To fix this error, you should ensure that the input to the layer is a tensor. You can use the `tf.constant` function to create a tensor from a Python list or NumPy array:
```
import tensorflow as tf
# create a tensor from a Python list
input_data = tf.constant([1, 2, 3, 4])
# create a tensor from a NumPy array
import numpy as np
input_data = tf.constant(np.array([[1, 2], [3, 4]]))
```
Once you have created a tensor, you can pass it as input to a Keras layer. For example:
```
from keras.layers import Dense
model = Sequential()
model.add(Dense(64, activation='relu', input_shape=(input_dim,)))
```
Here, the `Dense` layer takes the tensor `input_data` as input, and specifies the shape of the input tensor using the `input_shape` argument.
Inputs to a layer should be tensors. Got: <keras.layers.convolutional.conv1d.Conv1D object at 0x0000025F1DA0D340>
This error occurs when you try to pass a Keras layer object (in this case, a Conv1D layer) as an input to a function or another layer that expects a tensor as input.
To resolve this error, you need to pass a tensor as input to the function or layer instead of the Keras layer object. You can either define an input layer using the Input() function in Keras or use a previously defined layer that outputs a tensor as input to the current layer.
For example, if you have a Conv1D layer and want to pass its output as input to a Dense layer, you can do the following:
```
input_layer = Input(shape=(input_shape))
conv_layer = Conv1D(filters=32, kernel_size=3)(input_layer)
dense_layer = Dense(units=64)(conv_layer)
```
Here, we first define an input layer using the Input() function, then pass it to the Conv1D layer. We then pass the output tensor of the Conv1D layer as input to the Dense layer.
相关推荐
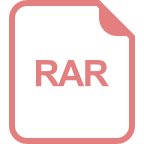
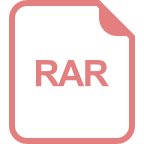
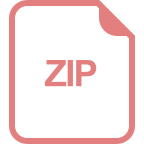












