private DatagramSocket socket; public Sender(DatagramSocket socket) { this.socket=socket; }的作用
时间: 2023-12-15 13:05:10 浏览: 30
这段代码定义了一个名为`Sender`的类,其中包含一个构造函数`Sender(DatagramSocket socket)`。构造函数接收一个`DatagramSocket`类型的参数,并将其赋值给类中的成员变量`socket`。
`DatagramSocket`是Java中用于实现数据报式Socket的类,因此这段代码的作用是:创建了一个`Sender`对象时,需要传入一个`DatagramSocket`类型的参数,以便在类的其他方法中使用该socket进行数据报的发送操作。
相关问题
java编写一个基于UDP的能够两人对聊的简单聊天程序
好的,以下是一个基于UDP的简单聊天程序的Java代码:
```java
import java.io.*;
import java.net.*;
public class Chat {
public static void main(String[] args) throws IOException {
// 创建两个UDP Socket
DatagramSocket socket1 = new DatagramSocket();
DatagramSocket socket2 = new DatagramSocket(9999);
// 创建两个线程,分别用于发送和接收消息
Thread sender = new Thread(new Sender(socket1, InetAddress.getLocalHost(), 8888));
Thread receiver = new Thread(new Receiver(socket2));
// 启动线程
sender.start();
receiver.start();
}
}
class Sender implements Runnable {
private DatagramSocket socket;
private InetAddress address;
private int port;
public Sender(DatagramSocket socket, InetAddress address, int port) {
this.socket = socket;
this.address = address;
this.port = port;
}
public void run() {
try {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
String message;
while (true) {
// 读取用户输入的消息
message = reader.readLine();
// 将消息转换为字节数组
byte[] data = message.getBytes();
// 创建一个DatagramPacket对象,包含要发送的数据、目的地地址和端口号
DatagramPacket packet = new DatagramPacket(data, data.length, address, port);
// 发送消息
socket.send(packet);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
class Receiver implements Runnable {
private DatagramSocket socket;
public Receiver(DatagramSocket socket) {
this.socket = socket;
}
public void run() {
try {
byte[] buffer = new byte[1024];
while (true) {
// 创建一个空的DatagramPacket对象,用于接收数据
DatagramPacket packet = new DatagramPacket(buffer, buffer.length);
// 接收消息
socket.receive(packet);
// 将接收到的数据转换为字符串
String message = new String(packet.getData(), 0, packet.getLength());
// 输出接收到的消息
System.out.println("对方:" + message);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
该程序创建了两个UDP Socket,一个用于发送消息,一个用于接收消息。在发送消息时,程序读取用户输入的消息,将其转换为字节数组并发送到指定的地址和端口号;在接收消息时,程序创建一个空的DatagramPacket对象,用于接收数据,并将其转换为字符串输出。
注意:该程序只是一个简单的示例,没有做太多的错误处理和安全处理,实际应用中还需要根据具体需求进行完善。
编写多线程的UDP网络程序: 编写数据接收类ReceiveThread,该类实现了Runnable接口,重写run()方法实现不断接受客户端发送数据的功能;编写数据发送类SendThread,该类同样实现了SendThread接口,重写run()方法实现通过键盘录入数据,将数据向接收端发送的功能。
好的,我能够为您提供一些多线程UDP网络编程的代码示例,帮助您实现您的需求。
首先,我们需要编写一个接收数据的类 `ReceiveThread`,它实现了 `Runnable` 接口来实现多线程。以下是代码示例:
```java
import java.net.*;
import java.io.*;
public class ReceiveThread implements Runnable {
private DatagramSocket socket;
private byte[] buffer;
public ReceiveThread(DatagramSocket socket, int bufferSize) {
this.socket = socket;
buffer = new byte[bufferSize];
}
@Override
public void run() {
while (true) {
try {
DatagramPacket packet = new DatagramPacket(buffer, buffer.length);
socket.receive(packet);
String received = new String(packet.getData(), 0, packet.getLength());
System.out.println("Received: " + received);
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
然后,我们需要编写一个发送数据的类 `SendThread`,它同样实现了 `Runnable` 接口来实现多线程。以下是代码示例:
```java
import java.net.*;
import java.io.*;
public class SendThread implements Runnable {
private DatagramSocket socket;
private InetAddress address;
private int port;
public SendThread(DatagramSocket socket, InetAddress address, int port) {
this.socket = socket;
this.address = address;
this.port = port;
}
@Override
public void run() {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
while (true) {
try {
String message = reader.readLine();
byte[] buffer = message.getBytes();
DatagramPacket packet = new DatagramPacket(buffer, buffer.length, address, port);
socket.send(packet);
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
最后,在主程序中,我们需要创建两个线程,一个用于接收数据,另一个用于发送数据。以下是代码示例:
```java
import java.net.*;
public class Main {
public static void main(String[] args) {
try {
DatagramSocket socket = new DatagramSocket(12345);
// 创建接收数据线程
ReceiveThread receiveThread = new ReceiveThread(socket, 1024);
Thread receiver = new Thread(receiveThread);
receiver.start();
// 创建发送数据线程
InetAddress address = InetAddress.getByName("localhost");
SendThread sendThread = new SendThread(socket, address, 54321);
Thread sender = new Thread(sendThread);
sender.start();
} catch (SocketException | UnknownHostException e) {
e.printStackTrace();
}
}
}
```
在这个示例中,我们创建了一个 `DatagramSocket` 对象来接收和发送数据。在主程序中,我们创建了两个线程,一个用于接收数据,另一个用于发送数据。其中,接收数据的线程会一直循环等待客户端发送数据,而发送数据的线程会通过键盘录入获取用户输入的数据,并将数据发送给接收端。
希望这个示例对您有所帮助。
相关推荐
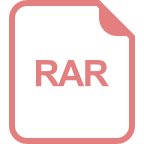
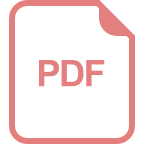
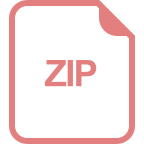












