linux C语言实现发送get请求,并打印回复消息
时间: 2024-04-03 11:35:20 浏览: 17
好的,我可以帮你解答这个问题。
在 Linux 下,可以使用 libcurl 库来发送 HTTP 请求并获取响应。以下是一个简单的示例代码,用于发送 GET 请求并打印响应消息:
```c
#include <stdio.h>
#include <curl/curl.h>
size_t write_callback(char *ptr, size_t size, size_t nmemb, void *userdata)
{
printf("%.*s", (int)(size * nmemb), ptr);
return size * nmemb;
}
int main()
{
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if (curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com");
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L);
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, write_callback);
res = curl_easy_perform(curl);
if (res != CURLE_OK)
fprintf(stderr, "curl_easy_perform() failed: %s\n", curl_easy_strerror(res));
curl_easy_cleanup(curl);
}
return 0;
}
```
这个程序中,我们首先初始化了一个 CURL 对象,然后设置了要请求的 URL,并打开了自动重定向选项,以便能够跟随重定向。接着,我们设置一个回调函数,用于处理响应消息,最后调用 `curl_easy_perform()` 函数来执行请求并获取响应。
回调函数 `write_callback()` 接收 4 个参数,分别是数据指针、数据块大小、数据块数量和一个用户数据指针。在这个函数中,我们只是简单地打印出了收到的数据。如果你需要对响应数据进行其他处理,可以在这个函数中进行。
注意,这个代码中没有做任何错误处理,实际使用时需要根据具体情况进行处理。另外,需要在编译时链接 libcurl 库,例如使用以下命令进行编译:
```
gcc -o example example.c -lcurl
```
相关推荐
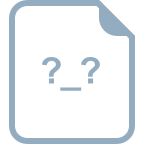
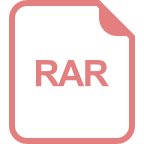














