C++二叉树平衡树算法实现 要求实现1.能够针对给定的输入序列,建立其存储结构 2.根据1的存储结构,实现各种遍历 3.对于生成的二叉平衡树,能实现数据的查找,插入,删除 4.对生成的树,能输出各种遍历结果
时间: 2024-03-25 13:40:09 浏览: 73
好的,我会尽力回答您的问题。首先,建议您使用AVL树作为二叉平衡树的实现,因为它比红黑树的实现更简单。
以下是C++实现AVL树的基本步骤:
1. 定义结点结构体
```c++
struct Node {
int val, height;
Node *left, *right;
Node(int v) : val(v), height(1), left(nullptr), right(nullptr) {}
};
```
2. 实现插入操作
```c++
Node* insert(Node* root, int val) {
if (root == nullptr) {
return new Node(val);
}
if (val < root->val) {
root->left = insert(root->left, val);
} else if (val > root->val) {
root->right = insert(root->right, val);
} else {
return root; // 值已经存在,不需要插入
}
int balance = getBalance(root);
if (balance > 1 && val < root->left->val) { // LL型
return rightRotate(root);
}
if (balance < -1 && val > root->right->val) { // RR型
return leftRotate(root);
}
if (balance > 1 && val > root->left->val) { // LR型
root->left = leftRotate(root->left);
return rightRotate(root);
}
if (balance < -1 && val < root->right->val) { // RL型
root->right = rightRotate(root->right);
return leftRotate(root);
}
root->height = 1 + max(getHeight(root->left), getHeight(root->right));
return root;
}
```
3. 实现删除操作
```c++
Node* deleteNode(Node* root, int val) {
if (root == nullptr) {
return root;
}
if (val < root->val) {
root->left = deleteNode(root->left, val);
} else if (val > root->val) {
root->right = deleteNode(root->right, val);
} else {
if (root->left == nullptr || root->right == nullptr) {
Node* temp = root->left ? root->left : root->right;
if (temp == nullptr) {
temp = root;
root = nullptr;
} else {
*root = *temp;
delete temp;
}
} else {
Node* temp = minValueNode(root->right);
root->val = temp->val;
root->right = deleteNode(root->right, temp->val);
}
}
if (root == nullptr) {
return root;
}
root->height = 1 + max(getHeight(root->left), getHeight(root->right));
int balance = getBalance(root);
if (balance > 1 && getBalance(root->left) >= 0) { // LL型
return rightRotate(root);
}
if (balance < -1 && getBalance(root->right) <= 0) { // RR型
return leftRotate(root);
}
if (balance > 1 && getBalance(root->left) < 0) { // LR型
root->left = leftRotate(root->left);
return rightRotate(root);
}
if (balance < -1 && getBalance(root->right) > 0) { // RL型
root->right = rightRotate(root->right);
return leftRotate(root);
}
return root;
}
```
4. 实现遍历操作
```c++
// 中序遍历
void inorder(Node* root) {
if (root == nullptr) {
return;
}
inorder(root->left);
cout << root->val << " ";
inorder(root->right);
}
// 先序遍历
void preorder(Node* root) {
if (root == nullptr) {
return;
}
cout << root->val << " ";
preorder(root->left);
preorder(root->right);
}
// 后序遍历
void postorder(Node* root) {
if (root == nullptr) {
return;
}
postorder(root->left);
postorder(root->right);
cout << root->val << " ";
}
```
5. 实现其它辅助操作
```c++
// 获取结点高度
int getHeight(Node* root) {
return root == nullptr ? 0 : root->height;
}
// 获取结点平衡因子
int getBalance(Node* root) {
return root == nullptr ? 0 : getHeight(root->left) - getHeight(root->right);
}
// 左旋转
Node* leftRotate(Node* x) {
Node* y = x->right;
Node* z = y->left;
y->left = x;
x->right = z;
x->height = 1 + max(getHeight(x->left), getHeight(x->right));
y->height = 1 + max(getHeight(y->left), getHeight(y->right));
return y;
}
// 右旋转
Node* rightRotate(Node* y) {
Node* x = y->left;
Node* z = x->right;
x->right = y;
y->left = z;
y->height = 1 + max(getHeight(y->left), getHeight(y->right));
x->height = 1 + max(getHeight(x->left), getHeight(x->right));
return x;
}
// 获取最小值结点
Node* minValueNode(Node* root) {
Node* current = root;
while (current->left != nullptr) {
current = current->left;
}
return current;
}
```
以上就是基本的实现代码,您可以根据您的具体需求进行修改和扩展。希望对您有所帮助!
阅读全文
相关推荐
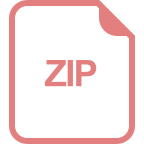
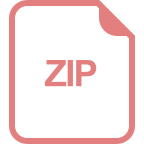
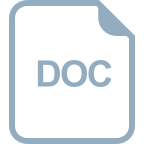
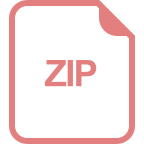
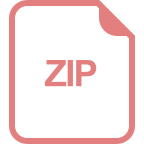
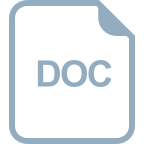
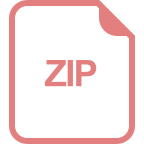
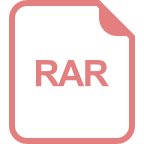
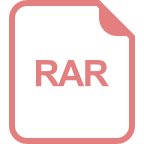
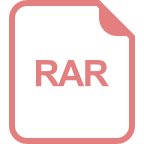
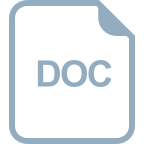
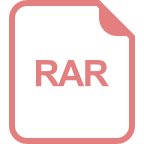
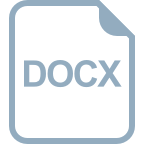
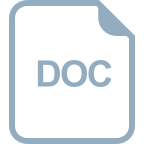
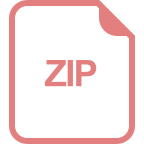
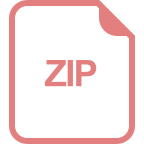
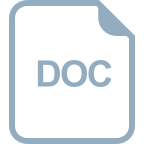