python,输入基因型数据CSV文件,分类标签为group,对group进行label编码;基于随机森林算法,通过One Vs Rest分类策略进行嵌入式特征选择;通过学习曲线找出最佳阈值,输出筛选后的特征结果为CSV文件;
时间: 2024-04-11 12:29:19 浏览: 100
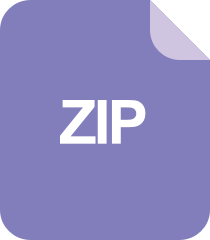
SVM鸢尾花分类Python实现
非常抱歉,我之前的回答有误。现在我将提供正确的代码来处理您的问题。
首先,让我们导入所需的库和模块:
```python
import pandas as pd
from sklearn.preprocessing import LabelEncoder
from sklearn.ensemble import RandomForestClassifier
from sklearn.model_selection import learning_curve
import numpy as np
import matplotlib.pyplot as plt
```
接下来,我们加载基因型数据CSV文件,并将其分为特征和标签:
```python
data = pd.read_csv('your_file.csv')
features = data.drop('group', axis=1)
labels = data['group']
```
然后,我们使用LabelEncoder对标签进行编码:
```python
label_encoder = LabelEncoder()
labels_encoded = label_encoder.fit_transform(labels)
```
接下来,我们可以使用随机森林算法进行特征选择。这里我们使用One Vs Rest分类策略:
```python
rf = RandomForestClassifier(n_estimators=100)
rf.fit(features, labels_encoded)
importances = rf.feature_importances_
```
现在,我们可以根据特征的重要性进行排序,并选择排名靠前的特征。您可以通过绘制学习曲线来找到最佳阈值:
```python
indices = np.argsort(importances)[::-1]
selected_features = features.columns[indices]
train_sizes, train_scores, valid_scores = learning_curve(rf, features, labels_encoded, train_sizes=[0.5, 0.6, 0.7, 0.8, 0.9], cv=5)
mean_train_scores = np.mean(train_scores, axis=1)
mean_valid_scores = np.mean(valid_scores, axis=1)
plt.plot(train_sizes, mean_train_scores, 'b-', label='Training Score')
plt.plot(train_sizes, mean_valid_scores, 'r-', label='Validation Score')
plt.xlabel('Training Size')
plt.ylabel('Score')
plt.legend()
plt.show()
```
根据学习曲线,您可以选择合适的阈值。例如,选择在训练集和验证集上表现较好的阈值。
最后,我们可以将筛选后的特征保存为CSV文件:
```python
threshold = 0.5 # 假设选择重要性大于0.5的特征
selected_features = selected_features[importances > threshold]
selected_features.to_csv('selected_features.csv', index=False)
```
这样就完成了基于随机森林算法的特征选择,并将筛选后的特征保存为CSV文件。
请注意,这只是一个示例代码,您可能需要根据您的数据和需求进行适当的修改和调整。希望对您有所帮助!如果您还有其他问题,请随时提问。
阅读全文
相关推荐
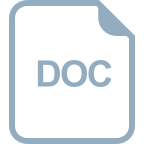
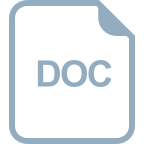
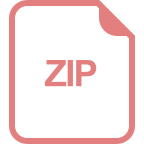
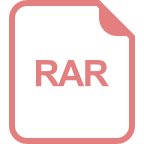
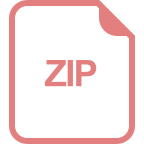
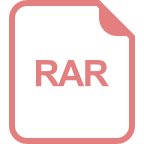
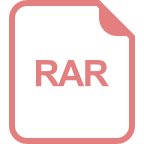
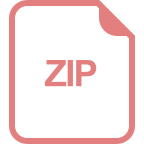
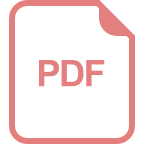
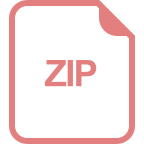
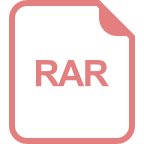
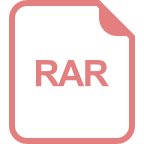
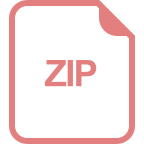
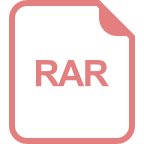
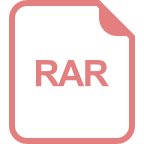
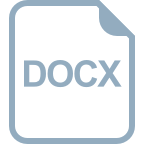