Python太阳系螺旋线运动
时间: 2023-12-05 08:40:49 浏览: 128
以下是Python太阳系螺旋线运动的代码和解释:
```python
import turtle
import math
# 定义行星类
class Planet:
def __init__(self, name, radius, distance, speed, color):
self.name = name # 行星名称
self.radius = radius # 行星半径
self.distance = distance # 行星距离太阳的距离
self.speed = speed # 行星公转速度
self.color = color # 行星颜色
# 定义太阳系类
class SolarSystem:
def __init__(self):
self.planets = [] # 行星列表
# 添加行星
def add_planet(self, planet):
self.planets.append(planet)
# 运行太阳系
def run(self):
# 初始化画布
turtle.bgcolor("black")
turtle.setup(800, 800)
turtle.title("Solar System")
# 循环绘制行星
while True:
for planet in self.planets:
# 计算行星当前位置
planet.angle += planet.speed
x = planet.distance * math.cos(math.radians(planet.angle))
y = planet.distance * math.sin(math.radians(planet.angle))
# 绘制行星
turtle.penup()
turtle.goto(x, y)
turtle.pendown()
turtle.dot(planet.radius, planet.color)
# 更新画布
turtle.update()
# 创建太阳系
solar_system = SolarSystem()
# 添加行星
sun = Planet("Sun", 50, 0, 0, "yellow")
mercury = Planet("Mercury", 5, 100, 5, "gray")
venus = Planet("Venus", 10, 150, 3, "orange")
earth = Planet("Earth", 15, 200, 2, "blue")
mars = Planet("Mars", 10, 250, 1, "red")
jupiter = Planet("Jupiter", 30, 300, 0.5, "brown")
saturn = Planet("Saturn", 25, 350, 0.3, "pink")
uranus = Planet("Uranus", 20, 400, 0.2, "light blue")
neptune = Planet("Neptune", 15, 450, 0.1, "purple")
solar_system.add_planet(sun)
solar_system.add_planet(mercury)
solar_system.add_planet(venus)
solar_system.add_planet(earth)
solar_system.add_planet(mars)
solar_system.add_planet(jupiter)
solar_system.add_planet(saturn)
solar_system.add_planet(uranus)
solar_system.add_planet(neptune)
# 运行太阳系
solar_system.run()
```
代码解释:
1. 首先定义了一个行星类Planet,包含行星名称、半径、距离太阳的距离、公转速度和颜色等属性。
2. 然后定义了一个太阳系类SolarSystem,包含一个行星列表,可以添加行星和运行太阳系。
3. 在运行太阳系的过程中,循环绘制每个行星。对于每个行星,计算其当前位置,然后在该位置绘制一个圆点表示行星。
4. 最后创建太阳系对象,添加各个行星,然后运行太阳系。
阅读全文
相关推荐
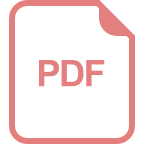
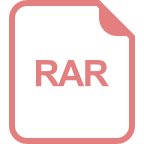
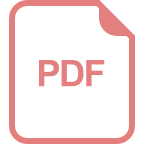
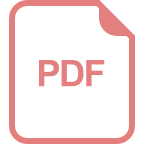
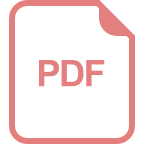
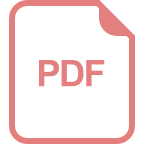
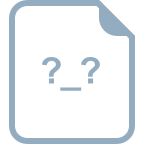
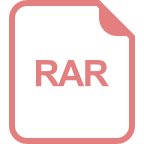
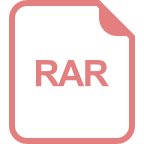
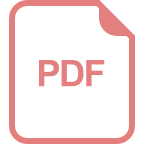