用c语言编写多个的同学的信息进行查询
时间: 2024-09-06 17:04:41 浏览: 60
在C语言中编写一个程序来查询多个同学的信息通常涉及以下几个步骤:
1. 定义一个结构体来存储每个同学的信息。这个结构体可能包括姓名、学号、年龄、性别等字段。
2. 创建一个数组或链表来存储多个同学的信息实例。
3. 编写函数来实现增加、删除、显示和查询等功能。
4. 实现一个查询功能,可以通过学号或者其他唯一标识来查找特定同学的信息。
下面是一个简化的示例代码,展示如何定义结构体、初始化同学信息数组以及实现一个基本的查询功能:
```c
#include <stdio.h>
#include <string.h>
// 定义学生信息结构体
typedef struct {
int id; // 学号
char name[50]; // 姓名
int age; // 年龄
char gender[10]; // 性别
} Student;
// 函数声明
void printStudentInfo(Student stu);
int findStudentIndexById(Student students[], int size, int id);
int main() {
// 创建一个学生信息数组
Student students[] = {
{1, "张三", 20, "男"},
{2, "李四", 21, "女"},
{3, "王五", 22, "男"}
};
int size = sizeof(students) / sizeof(students[0]); // 计算数组中元素的数量
// 查询学号为2的学生信息
int idToFind = 2;
int index = findStudentIndexById(students, size, idToFind);
if (index != -1) {
printStudentInfo(students[index]);
} else {
printf("未找到学号为%d的学生。\n", idToFind);
}
return 0;
}
// 打印学生信息的函数
void printStudentInfo(Student stu) {
printf("学号:%d\n姓名:%s\n年龄:%d\n性别:%s\n", stu.id, stu.name, stu.age, stu.gender);
}
// 根据学号查询学生在数组中的索引,如果未找到返回-1
int findStudentIndexById(Student students[], int size, int id) {
for (int i = 0; i < size; i++) {
if (students[i].id == id) {
return i;
}
}
return -1;
}
```
这个程序中,`findStudentIndexById` 函数用于在数组中查找具有特定学号的学生的索引。如果找到了,它会返回索引值;如果没有找到,它会返回 `-1`。然后可以使用这个索引来访问和打印学生的信息。
阅读全文
相关推荐
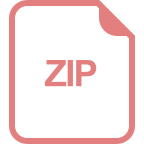
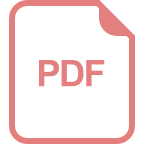
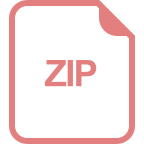

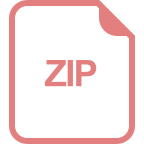
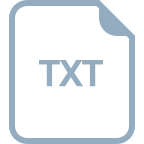
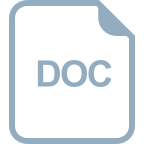
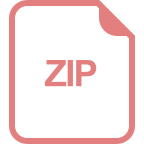
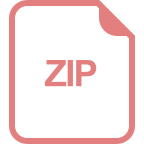
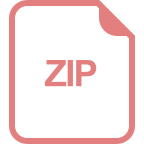
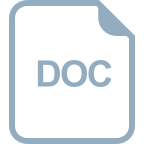
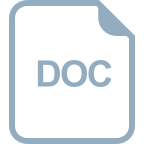
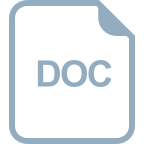
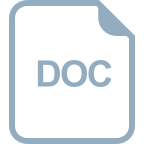
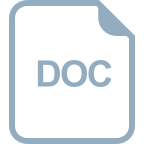
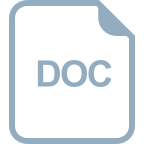


