opencv阈值分割算法c++
时间: 2023-11-07 10:38:21 浏览: 162
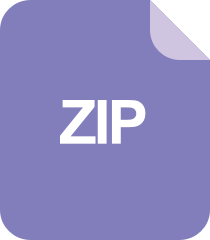
opencv-基于c++实现的opencv图像处理算法之自适应阈值分割算法.zip
Sure, I'd be happy to help you with that! The OpenCV library provides different thresholding methods for image segmentation in C language. The most common ones are binary, adaptive, and Otsu thresholding. Do you have any specific questions about these methods or their implementation in C?
阅读全文
相关推荐
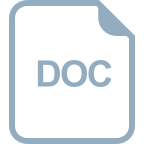
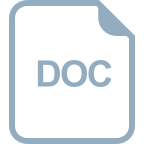
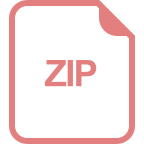
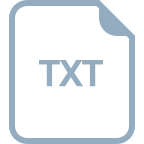
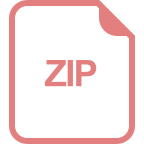
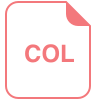

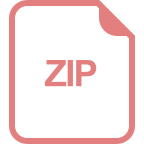
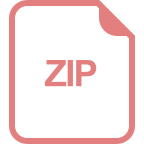
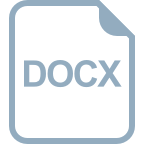



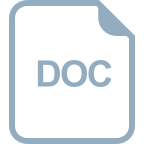
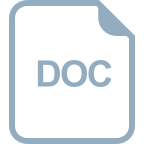