// 文件名 GreetingClient.java import java.net.*; import java.io.*; public class GreetingClient { public static void main(String [] args) { String serverName = args[0]; int port = Integer.parseInt(args[1]); try { System.out.println("连接到主机:" + serverName + " ,端口号:" + port); Socket client = new Socket(serverName, port); System.out.println("远程主机地址:" + client.getRemoteSocketAddress()); OutputStream outToServer = client.getOutputStream(); DataOutputStream out = new DataOutputStream(outToServer); out.writeUTF("Hello from " + client.getLocalSocketAddress()); InputStream inFromServer = client.getInputStream(); DataInputStream in = new DataInputStream(inFromServer); System.out.println("服务器响应: " + in.readUTF()); client.close(); }catch(IOException e) { e.printStackTrace(); } } }
时间: 2024-04-16 20:29:10 浏览: 80
这是一个简单的 Java 客户端程序,用于连接到指定的主机和端口。它首先创建一个 Socket 对象并连接到指定的服务器。然后,它通过输出流将一条消息发送给服务器。接下来,它从服务器接收响应并将其打印出来。最后,它关闭连接。
请注意,这个程序需要通过命令行参数传递服务器的主机名和端口号。你可以在命令行中运行这个程序,并将主机名和端口号作为参数传递给它。例如:
```shell
java GreetingClient example.com 1234
```
这将连接到主机 example.com 的端口 1234,并发送一条消息给服务器。然后,它将打印出服务器的响应。
相关问题
import java.io.FileInputStream; public class File { public static void main(String[] args) { File file = new File(pathname:"test.txt"); file.createNewFile();//创建文件 System.out.println(file.getName());//输入文件名 System.out.println(file.exists());//判断是否存在文件或者目录 System.out.println(file.isHidden()); System.out.println(file.isFile()); File file1=new File(pathname:"/Users/chen"); } }
你好!对于你的代码,我发现了一些错误。首先,在创建`File`对象时,你应该使用正确的构造函数来指定文件的路径,而不是使用`pathname`作为参数名。其次,在创建文件之前,你需要捕获可能抛出的`IOException`。最后,你可以使用`getAbsolutePath()`方法来获取文件的绝对路径。下面是修改后的代码:
```java
import java.io.File;
import java.io.IOException;
public class FileTest {
public static void main(String[] args) {
File file = new File("test.txt");
try {
if (file.createNewFile()) {
System.out.println("文件创建成功");
} else {
System.out.println("文件已存在");
}
} catch (IOException e) {
e.printStackTrace();
}
System.out.println(file.getName());//输入文件名
System.out.println(file.exists());//判断是否存在文件或者目录
System.out.println(file.isHidden());
System.out.println(file.isFile());
File file1 = new File("/Users/chen");
System.out.println(file1.getAbsolutePath());//获取文件的绝对路径
}
}
```
希望这可以帮助到你!如果你有任何其他问题,请随时问我。
import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; public class TextFileAnalyzer { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); // 获取文件名 System.out.print("请输入文件名:"); String fileName = scanner.nextLine(); // 打开文件 File file = new File(fileName); Scanner fileScanner; try { fileScanner = new Scanner(file); } catch (FileNotFoundException e) { System.out.println("文件不存在!"); return; } // 统计字符和字符串个数 int charCount = 0; int stringCount = 0; while (fileScanner.hasNextLine()) { String line = fileScanner.nextLine(); charCount += line.replaceAll("\\s", "").length(); stringCount += line.split("\\s+").length; } // 输出结果 System.out.printf("文件中字符个数:%d\n", charCount); System.out.printf("文件中字符串个数:%d\n", stringCount); // 关闭文件 fileScanner.close(); } }
这是一个 Java 代码,可以用于分析文本文件中字符和字符串的个数。程序首先会要求用户输入文件名,然后打开文件进行分析。程序使用 Scanner 对象读取文件内容,统计文件中字符的个数和字符串的个数,最后输出结果。
在循环中,程序使用 replaceAll() 方法去除字符串中的空白字符,然后计算剩下的字符的个数。程序还使用 split() 方法将字符串按照空白字符分割成字符串数组,然后计算数组的长度,即为字符串的个数。
需要注意的是,程序在打开文件时可能会发生 FileNotFoundException 异常,需要进行异常处理。另外,在使用完 Scanner 对象后,需要关闭文件。
阅读全文
相关推荐
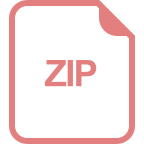
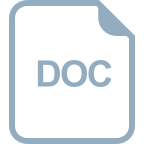
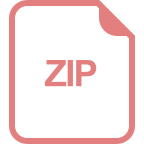














