回归模型——感知机 C++带类实现及案例
时间: 2023-10-25 16:10:13 浏览: 109
感知机是二分类的线性分类模型,其输入为实例的特征向量,输出为实例的类别,取 +1 和 -1 二值。感知机对应于输入空间(特征空间)中将实例划分为正负两类的分离超平面,属于判别模型。
感知机学习算法是基于随机梯度下降法的。具体地,首先任意选取一个超平面,然后用梯度下降法不断地极小化目标函数,找出最优超平面。
以下是感知机算法的C++类实现及案例代码:
```c++
#include <iostream>
#include <vector>
#include <random>
using namespace std;
class Perceptron {
public:
Perceptron(int feature_num) : w(feature_num), b(0) {}
void train(const vector<vector<double>>& X, const vector<double>& y, int max_iter = 100) {
int n_samples = X.size();
int n_features = X[0].size();
mt19937 rng(0);
uniform_int_distribution<int> dist(0, n_samples - 1);
for (int iter = 0; iter < max_iter; iter++) {
int i = dist(rng);
double wx = 0;
for (int j = 0; j < n_features; j++) {
wx += X[i][j] * w[j];
}
double yi = y[i];
if (yi * (wx + b) <= 0) {
for (int j = 0; j < n_features; j++) {
w[j] += yi * X[i][j];
}
b += yi;
}
}
}
double predict(const vector<double>& x) {
double wx = 0;
int n_features = x.size();
for (int i = 0; i < n_features; i++) {
wx += x[i] * w[i];
}
return wx + b > 0 ? 1 : -1;
}
void print_weights() const {
cout << "w = [";
for (double wi : w) {
cout << wi << ", ";
}
cout << "], b = " << b << endl;
}
private:
vector<double> w;
double b;
};
int main() {
vector<vector<double>> X{
{3, 3},
{4, 3},
{1, 1}
};
vector<double> y{1, 1, -1};
Perceptron model(X[0].size());
model.train(X, y);
model.print_weights();
cout << "predict([3, 4]) = " << model.predict({3, 4}) << endl;
return 0;
}
```
在上述代码中,Perceptron类代表感知机模型。train函数接受训练数据X和y,以及最大迭代次数max_iter,默认为100。predict函数接受一个样本特征向量x,返回其预测的类别标签。print_weights函数打印训练后得到的权重和偏置项。
本例中,使用学习率为1的随机梯度下降法进行模型训练。训练数据X是一个3x2的矩阵,y是一个包含3个元素的向量,表示3个样本的类别标签。模型训练完毕后,使用predict函数对特定样本进行预测。
以上是感知机算法的C++类实现及案例代码,希望对你有所帮助。
阅读全文
相关推荐
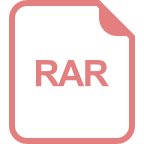
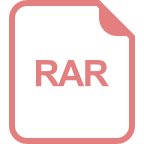
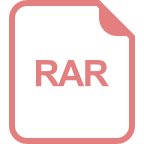


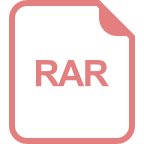