vue3 elementPlus 关闭弹窗再打开,弹窗中的子组件不展示
时间: 2024-02-21 16:02:01 浏览: 28
这个问题可能是由于弹窗关闭时,子组件并没有被销毁导致的。你可以在弹窗关闭时手动将子组件销毁,以确保下次打开弹窗时子组件能够正确展示。具体方法如下:
1. 给弹窗组件添加一个 `beforeDestroy` 钩子函数,在该钩子函数中手动将子组件销毁,例如:
```javascript
beforeDestroy() {
this.$refs.childComponent.$destroy();
}
```
2. 在打开弹窗的方法中,每次都重新创建一个子组件实例并将其挂载到弹窗中,例如:
```javascript
openDialog() {
const ChildComponent = Vue.extend(ChildComponent);
const childComponent = new ChildComponent().$mount();
this.$refs.dialog.$el.appendChild(childComponent.$el);
this.$refs.dialog.open();
}
```
这样做可以确保每次打开弹窗时都会创建一个新的子组件实例,并将其正确挂载到弹窗中。
相关问题
vue3 elementplus实现弹窗
使用 Element Plus 实现弹窗的步骤如下:
1. 安装 Element Plus
```bash
npm install element-plus --save
```
2. 在项目中引入 Element Plus
```javascript
import { ElButton, ElDialog } from 'element-plus';
import 'element-plus/lib/theme-chalk/index.css';
export default {
components: {
ElButton,
ElDialog
}
}
```
3. 在页面中使用 ElButton 和 ElDialog 组件
```html
<template>
<div>
<el-button @click="dialogVisible = true">打开弹窗</el-button>
<el-dialog title="提示" :visible.sync="dialogVisible">
<span>这是一段信息</span>
<div slot="footer">
<el-button @click="dialogVisible = false">取消</el-button>
<el-button type="primary" @click="submit">确定</el-button>
</div>
</el-dialog>
</div>
</template>
<script>
export default {
data() {
return {
dialogVisible: false
}
},
methods: {
submit() {
// 确定按钮的点击事件
}
}
}
</script>
```
4. 根据需要自定义弹窗的样式和行为。
以上就是使用 Element Plus 实现弹窗的基本步骤,可以根据自己的需求进行样式和行为的定制。
用vue3和elementplus写一段代码,要求能上传文件,并把文件名展示在弹窗中
好的,以下是符合您要求的代码示例:
```html
<template>
<div>
<el-button @click="dialogVisible = true">上传文件</el-button>
<el-dialog :visible.sync="dialogVisible" title="上传文件" width="30%">
<el-upload
class="upload-demo"
action="https://www.mocky.io/v2/5cc8019d300000980a055e76"
:on-success="handleSuccess"
:before-upload="beforeUpload"
:auto-upload="false"
>
<el-button slot="trigger" size="small" type="primary">选取文件</el-button>
<el-progress
class="progress"
:stroke-width="4"
:percentage="uploadPercent"
:color="uploadPercent === 100 ? '#00bfff' : '#409eff'"
:status="uploadPercent === 100 ? 'success' : ''"
:text-inside="true"
/>
<div slot="tip" class="el-upload__tip">只能上传jpg/png文件,且不超过500kb</div>
</el-upload>
<div slot="footer" class="dialog-footer">
<el-button @click="dialogVisible = false">取 消</el-button>
<el-button type="primary" :disabled="!uploadDone" @click="submitForm">确 定</el-button>
</div>
</el-dialog>
</div>
</template>
<script>
import { ref } from 'vue'
import { ElUpload, ElButton, ElDialog, ElProgress } from 'element-plus'
export default {
components: { ElUpload, ElButton, ElDialog, ElProgress },
setup() {
const dialogVisible = ref(false)
const uploadDone = ref(false)
const uploadPercent = ref(0)
const fileName = ref('')
const beforeUpload = (file) => {
const isJPG = file.type === 'image/jpeg' || file.type === 'image/png'
const isLt500K = file.size / 1024 < 500
if (!isJPG) {
this.$message.error('上传文件只能是 JPG/PNG 格式!')
return false
}
if (!isLt500K) {
this.$message.error('上传文件大小不能超过 500KB!')
return false
}
return true
}
const handleSuccess = (response, file) => {
uploadDone.value = true
fileName.value = file.name
}
const submitForm = () => {
console.log('提交文件:', fileName.value)
dialogVisible.value = false
}
return {
dialogVisible,
uploadDone,
uploadPercent,
beforeUpload,
handleSuccess,
submitForm,
fileName
}
}
}
</script>
```
这段代码使用了Element Plus中的`el-upload`组件实现文件上传功能,上传成功后会将`uploadDone`和`fileName`的值进行更新。弹窗的展示使用了`el-dialog`组件,其中`visible`属性通过`v-model`与`dialogVisible`变量进行双向绑定。最后,当用户点击确定按钮时,会将文件名传递给后端进行处理。
相关推荐
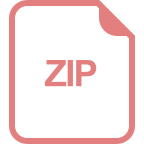
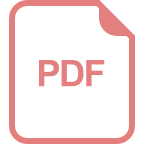
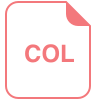
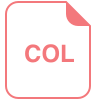
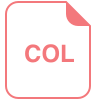
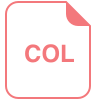
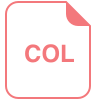







