利用另一个窗体的textbox的输入,将数据写入到datagridview中,textbox内容依次为任务名称、保养级别、保养内容、保养时长、保养角色
时间: 2023-11-27 21:03:45 浏览: 41
你可以通过以下代码实现:
1. 在datagridview中添加5个列,分别对应任务名称、保养级别、保养内容、保养时长、保养角色。
2. 在窗体加载时,将textbox绑定到另一个窗体的textbox的TextChanged事件,如下:
``` c#
private void Form1_Load(object sender, EventArgs e)
{
otherForm.textBox1.TextChanged += new EventHandler(otherTextBox_TextChanged);
}
void otherTextBox_TextChanged(object sender, EventArgs e)
{
string[] data = otherForm.textBox1.Text.Split(',');
dataGridView1.Rows.Add(data);
}
```
3. 在otherTextBox_TextChanged事件中,将另一个窗体的textbox的内容按逗号分隔成一个string数组,然后将数组内容添加到datagridview中的新行。
注意:otherForm是你打开另一个窗体的实例,textBox1是另一个窗体的textbox控件。另外,你需要考虑输入数据的合法性和处理错误情况。
相关问题
利用另一个窗体的textbox的输入,将数据写入到datagridview中
假设您的主窗体中有一个按钮,点击该按钮会打开一个新窗体,该新窗体中有一个 TextBox 和一个按钮,您可以按照以下步骤将 TextBox 中的数据写入到主窗体的 DataGridView 中:
1. 在主窗体中,为 DataGridView 绑定数据源(可以是 DataTable 或任何实现了 IList 接口的集合类)。
```csharp
private void Form1_Load(object sender, EventArgs e)
{
// 创建 DataTable 对象
DataTable table = new DataTable();
table.Columns.Add("姓名");
table.Columns.Add("年龄");
// 绑定数据源
dataGridView1.DataSource = table;
}
```
2. 在新窗体中,添加一个公共属性,用于获取 TextBox 中的数据。
```csharp
public partial class Form2 : Form
{
// 公共属性,用于获取 TextBox 中的数据
public string InputText
{
get { return textBox1.Text; }
}
public Form2()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
// 点击按钮时,关闭窗体
this.Close();
}
}
```
3. 在主窗体中,添加打开新窗体的按钮的事件处理程序,通过该事件处理程序打开新窗体,并将 TextBox 中的数据写入到 DataGridView 中。
```csharp
private void button1_Click(object sender, EventArgs e)
{
// 创建新窗体对象
Form2 form2 = new Form2();
// 显示新窗体
form2.ShowDialog();
// 获取新窗体中的数据
string inputText = form2.InputText;
// 将数据写入到 DataGridView 中
DataTable table = dataGridView1.DataSource as DataTable;
table.Rows.Add(inputText, 0);
}
```
上述代码中,我们首先创建了一个新窗体对象,并显示该窗体。在新窗体中输入数据并点击按钮后,新窗体会关闭,并将 TextBox 中的数据通过公共属性传递给主窗体。主窗体接收到数据后,将其添加到 DataGridView 的数据源中。请注意,我们在将数据添加到 DataTable 中时,将年龄设置为 0,因为我们没有在新窗体中输入年龄。如果您需要输入年龄,可以在新窗体中添加一个 TextBox,类似地获取数据并将其添加到 DataTable 中。
利用另一个窗体的textbox的输入,将多列数据写入到datagridview中
可以使用以下步骤实现:
1. 在需要获取输入的窗体中,将输入的数据存储到一个字符串或数组中。
2. 在需要写入数据的窗体中,打开对应的窗体并获取其中的字符串或数组。
3. 将获取到的数据进行解析,得到每一列的数据。
4. 将得到的数据逐行添加到DataGridView中。
以下是一个示例代码:
在窗体1中获取输入的数据:
```
private void btnOK_Click(object sender, EventArgs e)
{
string inputData = textBox1.Text;
Form2 form2 = new Form2(inputData);
form2.Show();
}
```
在窗体2中解析并添加数据到DataGridView中:
```
public Form2(string inputData)
{
InitializeComponent();
string[] rows = inputData.Split('\n');
foreach (string row in rows)
{
string[] cells = row.Trim().Split('\t');
dataGridView1.Rows.Add(cells);
}
}
```
注意:上述示例中假设输入的数据是以制表符分隔的多列数据,如果输入的数据格式不同,需要相应地修改解析数据的代码。
相关推荐














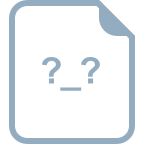
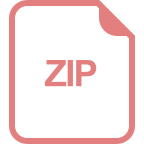